用Jquery实现一个表单,有用户名,密码,年龄及提交选项; 点击提交后, 在下面的表格中产生一条记录; 注意: 1. 初始会有一条表格头信息,只是在后面追加记录 2. 信息头为用户名,密码,年龄,操作 3. 点击删除操作, 可以删除指定记录
时间: 2023-09-13 10:10:17 浏览: 84
下面是使用 jQuery 实现的代码:
```html
<form>
<label>用户名:<input type="text" name="username"></label><br>
<label>密码:<input type="password" name="password"></label><br>
<label>年龄:<input type="number" name="age"></label><br>
<button type="button" id="submitBtn">提交</button>
</form>
<table>
<thead>
<tr>
<th>用户名</th>
<th>密码</th>
<th>年龄</th>
<th>操作</th>
</tr>
</thead>
<tbody id="recordList">
</tbody>
</table>
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
<script>
// 点击提交按钮时触发
$('#submitBtn').click(function() {
let username = $('input[name="username"]').val();
let password = $('input[name="password"]').val();
let age = $('input[name="age"]').val();
// 发送 POST 请求到 submit.php,将表单数据提交
$.post('submit.php', { username, password, age }, function(data) {
// 渲染表格中的记录
renderRecords(data);
});
});
// 渲染表格中的记录
function renderRecords(records) {
let tbody = $('#recordList');
tbody.empty(); // 清空表格内容
// 添加表头
let tr = $('<tr>').appendTo(tbody);
$('<th>').text('用户名').appendTo(tr);
$('<th>').text('密码').appendTo(tr);
$('<th>').text('年龄').appendTo(tr);
$('<th>').text('操作').appendTo(tr);
// 添加记录
for (let i = 0; i < records.length; i++) {
let record = records[i];
let tr = $('<tr>').appendTo(tbody);
$('<td>').text(record.username).appendTo(tr);
$('<td>').text(record.password).appendTo(tr);
$('<td>').text(record.age).appendTo(tr);
let td4 = $('<td>').appendTo(tr);
$('<button>').text('删除').click(function() {
// 发送 POST 请求到 delete.php,删除指定记录
$.post('delete.php', { index: i }, function(data) {
// 渲染表格中的记录
renderRecords(data);
});
}).appendTo(td4);
}
}
// 页面加载时,从 get.php 获取已有记录并渲染
$.get('get.php', function(data) {
renderRecords(data);
});
</script>
```
上面的代码中,我们使用了 jQuery 的 `$.post` 函数和 `$.get` 函数来发送 POST 和 GET 请求。`submit.php` 和 `delete.php` 的代码与上面使用 PHP 实现的代码相同,这里不再赘述。需要注意的是,在 `$.post` 函数和 `$.get` 函数中,第二个参数是提交的数据,可以使用对象字面量来简化代码。另外,`renderRecords` 函数也需要修改为接收参数。
阅读全文
相关推荐
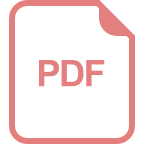

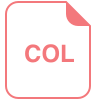
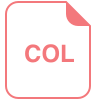
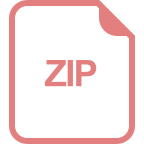
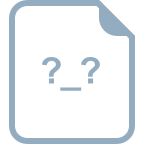
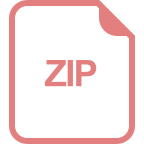
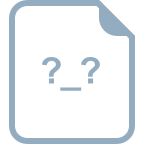
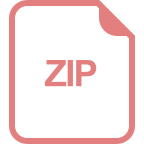
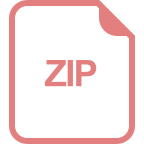