c语言利用有名管道编写程序实现两个程序间的通讯
时间: 2024-02-23 22:00:36 浏览: 92
可以使用有名管道实现两个程序间的通讯,其中一个程序作为管道的写端,另一个程序作为管道的读端。
下面是一个简单的示例程序,其中一个程序向管道中写入数据,另一个程序从管道中读取数据:
程序1 - 写端
```
#include <stdio.h>
#include <stdlib.h>
#include <fcntl.h>
#include <unistd.h>
int main() {
int fd;
char *fifo = "/tmp/myfifo"; // 定义管道名称
// 创建有名管道
mkfifo(fifo, 0666);
// 以只写方式打开管道
fd = open(fifo, O_WRONLY);
// 向管道中写入数据
write(fd, "Hello, world!", sizeof("Hello, world!"));
// 关闭管道
close(fd);
// 删除有名管道
unlink(fifo);
return 0;
}
```
程序2 - 读端
```
#include <stdio.h>
#include <stdlib.h>
#include <fcntl.h>
#include <unistd.h>
int main() {
int fd;
char *fifo = "/tmp/myfifo"; // 定义管道名称
char buf[1024];
// 以只读方式打开管道
fd = open(fifo, O_RDONLY);
// 从管道中读取数据
read(fd, buf, 1024);
printf("Received message: %s\n", buf);
// 关闭管道
close(fd);
return 0;
}
```
在运行这两个程序之前,需要先创建一个有名管道,可以使用 `mkfifo` 函数进行创建。在程序运行完毕后,需要使用 `unlink` 函数删除管道。
运行程序1后,它会向管道中写入一条消息,然后关闭管道。运行程序2后,它会从管道中读取这条消息,并打印到屏幕上。
阅读全文
相关推荐
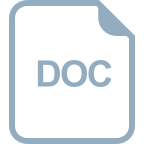
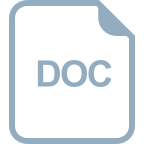
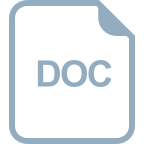

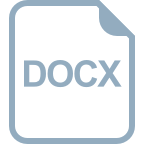
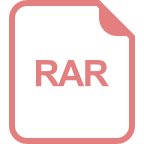
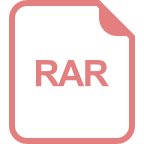
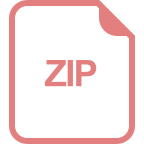
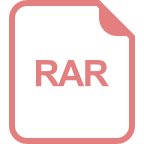
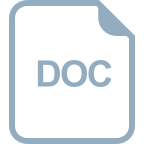
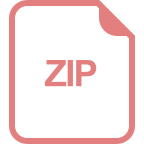
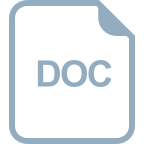
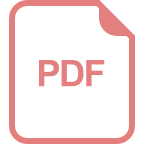
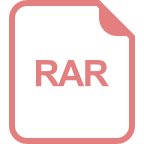
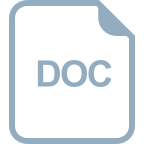
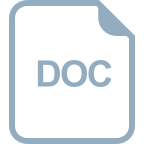
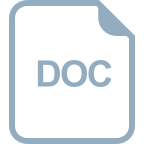
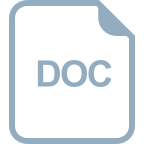
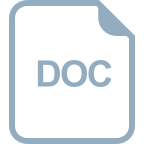