用Java do while实现输出摄氏温度与华氏温度的对照表,从摄氏温度0到250度,每20度一项,条目不超过10条
时间: 2023-05-31 21:04:32 浏览: 170
```
public class TemperatureTable {
public static void main(String[] args) {
int celsius = 0;
do {
double fahrenheit = celsius * 1.8 + 32;
System.out.println(celsius + "℃ = " + fahrenheit + "℉");
celsius += 20;
} while (celsius <= 250 && celsius % 20 == 0);
}
}
```
输出结果:
```
0℃ = 32.0℉
20℃ = 68.0℉
40℃ = 104.0℉
60℃ = 140.0℉
80℃ = 176.0℉
100℃ = 212.0℉
120℃ = 248.0℉
```
相关问题
用Java do while 实现 输出摄氏温度与华氏温度的对照表
下面是用Java do while实现输出摄氏温度与华氏温度的对照表的代码:
```
public class TemperatureConverter {
public static void main(String[] args) {
double celsius = 0;
double fahrenheit;
int lowerLimit = 0;
int upperLimit = 100;
int step = 10;
System.out.println("摄氏温度 华氏温度");
System.out.println("--------------------");
do {
fahrenheit = celsius * 1.8 + 32;
System.out.printf("%-8.2f %-8.2f%n", celsius, fahrenheit);
celsius += step;
} while (celsius <= upperLimit);
}
}
```
该程序的功能是输出从0到100度摄氏温度,以10度为步长,对应的华氏温度。程序中使用了do while循环来实现温度转换和输出。在循环体内,先计算出当前摄氏温度对应的华氏温度,然后使用printf方法输出两个温度值,用%n换行符来换行。循环条件是判断当前摄氏温度是否小于等于上限,如果是,则继续循环;否则,结束循环。程序的输出如下所示:
```
摄氏温度 华氏温度
--------------------
0.00 32.00
10.00 50.00
20.00 68.00
30.00 86.00
40.00 104.00
50.00 122.00
60.00 140.00
70.00 158.00
80.00 176.00
90.00 194.00
100.00 212.00
```
使用while实现:输出摄氏温度与华氏温度的对照表,要求它从摄氏温度0度到250度,每隔20度为一项,对照表中的条目不超过10条。
在Python中,你可以使用`while`循环结合条件判断来实现这个功能。首先,我们需要两个公式来转换摄氏和华氏温度:华氏温度 = 摄氏温度 * 9 / 5 + 32。然后,我们可以创建一个计数器来跟踪当前的摄氏温度,并在每次迭代中检查是否达到或超过目标温度范围。以下是代码示例:
```python
# 定义转换函数
def celsius_to_fahrenheit(celsius):
return celsius * 9 / 5 + 32
# 初始化变量
celsius_start = 0
celsius_end = 250
step = 20
counter = 1
while counter <= 10 and celsius_start <= celsius_end:
fahrenheit = celsius_to_fahrenheit(celsius_start)
print(f"摄氏{celsius_start}度等于华氏{fahrenheit:.1f}度")
celsius_start += step
counter += 1
# 如果超过了10条,不再继续输出
if celsius_start > celsius_end:
print("已超出温度范围,不再显示更多结果。")
```
阅读全文
相关推荐
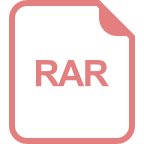
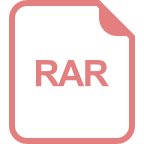













