decompose3 (Image, r, g, b)
时间: 2024-05-05 08:15:37 浏览: 75
As an AI language model, I'm not sure what exactly you want me to do with the given function name "decompose3 (Image, r, g, b)." However, based on my understanding, the function name suggests that it decomposes an image into its red, green, and blue channels.
Here is a possible implementation of such a function in Python:
```python
def decompose3(image):
r = image[:, :, 0] # extract the red channel
g = image[:, :, 1] # extract the green channel
b = image[:, :, 2] # extract the blue channel
return r, g, b # return the three channels as separate images
```
In this implementation, the function takes an image as input, which is assumed to be a NumPy array with three dimensions: height, width, and channel (RGB). The function then extracts the red, green, and blue channels from the image using NumPy array indexing, and returns them as three separate images.
You can use this function as follows:
```python
import matplotlib.pyplot as plt
from skimage import io
# load an example RGB image
image = io.imread('example.jpg')
# decompose the image into its red, green, and blue channels
r, g, b = decompose3(image)
# visualize the three channels separately
fig, (ax1, ax2, ax3) = plt.subplots(ncols=3, figsize=(10, 5))
ax1.imshow(r, cmap='Reds')
ax1.set_title('Red channel')
ax2.imshow(g, cmap='Greens')
ax2.set_title('Green channel')
ax3.imshow(b, cmap='Blues')
ax3.set_title('Blue channel')
plt.show()
```
This code loads an example RGB image using the skimage library, decomposes it into its three channels using the `decompose3` function we defined earlier, and then visualizes the three channels separately using Matplotlib. The resulting figure shows the red, green, and blue channels of the image side by side.
阅读全文
相关推荐
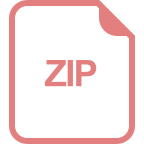
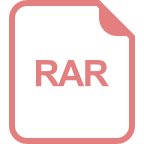
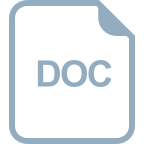















