python 写一个ping多个网络设备代码
时间: 2023-09-25 08:06:40 浏览: 179
### 回答1:
当然,我很乐意回答您的问题。以下是一个可以实现ping多个网络设备的Python代码示例:
```python
import os
def ping(ip):
"""
Ping 给定 IP,检查是否可以到达
"""
response = os.system("ping -n 1 " + ip)
return response == 0
if __name__ == '__main__':
devices = [
'192.168.1.1',
'192.168.1.2',
'192.168.1.3',
]
for device in devices:
if ping(device):
print(f'{device} is up!')
else:
print(f'{device} is down!')
```
在这个例子中,我们定义了一个`ping()`函数,它接受一个IP地址并返回True或False,表示是否能够到达该设备。然后我们定义了一个包含多个设备IP的列表,并使用一个循环来遍历它们并执行ping操作。如果ping成功,则输出“{设备} is up!”,否则输出“{设备} is down!”。
希望这个示例可以帮助您!
### 回答2:
以下是一个使用Python编写的ping多个网络设备的代码示例:
```python
import subprocess
def ping_device(device_ip):
# 使用subprocess模块执行ping命令
p = subprocess.Popen(['ping', '-c', '4', device_ip], stdout=subprocess.PIPE)
# 获取ping命令的输出结果
output, _ = p.communicate()
# 检查ping命令的返回值
if p.returncode == 0:
result = f"{device_ip} is reachable."
else:
result = f"{device_ip} is unreachable."
return result
def ping_multiple_devices(device_ips):
results = []
for device_ip in device_ips:
result = ping_device(device_ip)
results.append(result)
return results
# 要ping的设备IP列表
device_ips = ['192.168.0.1', '192.168.0.2', '192.168.0.3']
# 调用ping_multiple_devices函数并打印结果
ping_results = ping_multiple_devices(device_ips)
for result in ping_results:
print(result)
```
在上述代码中,我们首先定义了一个`ping_device`函数,用于ping单个设备。使用`subprocess.Popen`执行ping命令,并使用`communicate`方法获取ping命令的输出结果。根据ping命令的返回值(0表示成功,非0表示失败),我们可以判断设备是否可达,并返回相应结果。
然后,我们定义了`ping_multiple_devices`函数,该函数接收设备IP列表,并依次调用`ping_device`函数进行ping操作。最终,将每个设备的ping结果存储在一个列表中,并返回该列表。
最后,在主程序中定义了要ping的设备IP列表,并调用`ping_multiple_devices`函数进行ping操作。将结果打印出来。
这样,我们就可以使用这段代码快速、便捷地进行多个网络设备的ping操作。
### 回答3:
下面是一个简单的Python代码示例,可以使用ping命令来批量ping多个网络设备。
```python
import os
def ping_devices(devices):
for device in devices:
response = os.system("ping -c 1 " + device)
if response == 0:
print(device + " is online")
else:
print(device + " is offline")
# 定义需要ping的设备IP地址列表
devices = ["192.168.1.1", "192.168.1.2", "192.168.1.3"]
# 调用ping_devices函数
ping_devices(devices)
```
上述代码中,`ping_devices`函数接受一个设备IP地址列表作为参数,并使用`os.system`函数执行ping命令。如果ping命令返回值为0,表示设备在线;如果返回值为非零,则表示设备离线。根据返回值的不同,函数会打印出相应的设备在线或者离线的信息。
你可以根据自己的需求修改`devices`列表中的IP地址,添加或移除需要ping的设备。
阅读全文
相关推荐
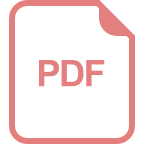
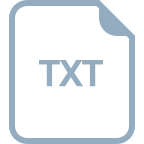
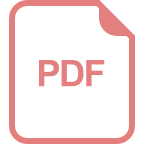
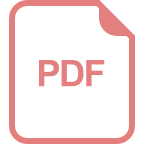
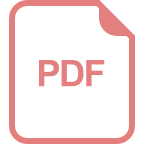
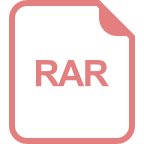
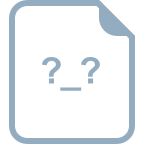
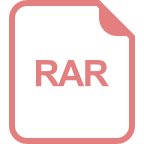
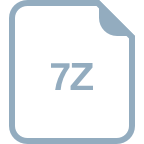
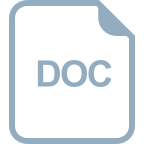
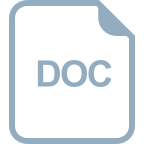
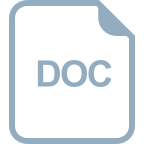
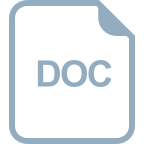
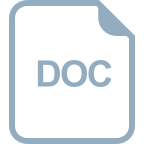
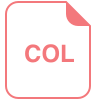
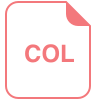
