编写linux 下c程序,以二进制形式创建,读写文件,检测文件是否存在,获取文件长度
时间: 2024-03-05 10:51:25 浏览: 54
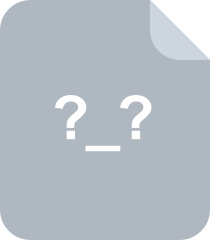
linux c 读写二进制文件
下面是一个简单的Linux下C语言程序,用于以二进制形式创建、读写文件,检测文件是否存在,获取文件长度:
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <fcntl.h>
#include <sys/stat.h>
int main(void) {
char* filename = "test.bin";
int fd;
struct stat filestat;
// 检查文件是否存在
if (access(filename, F_OK) == -1) {
printf("文件 %s 不存在\n", filename);
return 1;
}
// 以二进制形式打开文件
fd = open(filename, O_RDWR | O_BINARY);
if (fd == -1) {
perror("打开文件失败");
return 1;
}
// 获取文件长度
if (fstat(fd, &filestat) == -1) {
perror("获取文件长度失败");
return 1;
}
off_t filesize = filestat.st_size;
printf("文件长度为 %ld 字节\n", filesize);
// 写入数据
char* data = "Hello, World!";
if (write(fd, data, strlen(data)) != strlen(data)) {
perror("写入数据失败");
return 1;
}
// 读取数据
char buffer[1024];
lseek(fd, 0, SEEK_SET);
if (read(fd, buffer, filesize) != filesize) {
perror("读取数据失败");
return 1;
}
printf("读取到的数据为:%s\n", buffer);
// 关闭文件
if (close(fd) == -1) {
perror("关闭文件失败");
return 1;
}
return 0;
}
```
在程序中,我们首先使用`access()`函数检查文件是否存在,然后使用`open()`函数以二进制形式打开文件。接着,我们使用`fstat()`函数获取文件长度,并使用`write()`函数写入数据,使用`read()`函数读取数据。最后,我们使用`close()`函数关闭文件。
运行该程序时,需要将`test.bin`文件放在当前目录下。程序会检查文件是否存在,如果不存在则会输出错误信息并退出。如果文件存在,则会输出文件长度,并读取并输出文件中的数据。
阅读全文
相关推荐
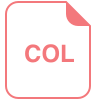
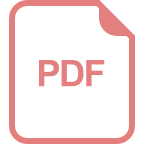
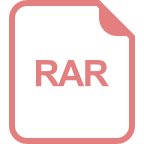
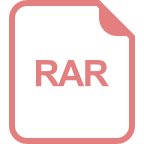
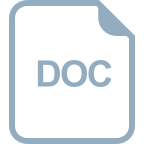
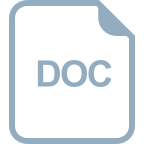
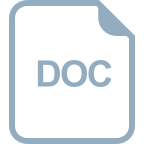
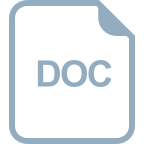
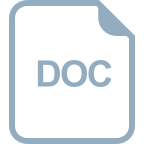
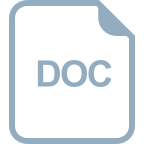
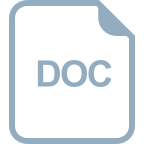
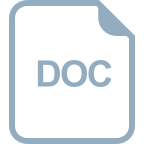
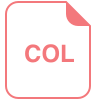
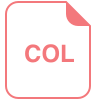
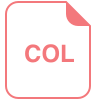