通过控制层编写出业务层代码 @ApiOperation(value = "根据ID获取用户信息") @ApiImplicitParams(value = { @ApiImplicitParam(name = "id", value = "用户ID", dataType = "Integer", dataTypeClass = Integer.class), }) @GetMapping("/select/id") public UserInfo selectUserInfoById(@RequestParam Integer id ) throws Exception { return deviceService.selectUserInfoById(id); }
时间: 2024-02-26 13:55:07 浏览: 89
这是一个使用Swagger2注解编写的Spring Boot接口,其中@ApiOperation表示接口的描述信息,@ApiImplicitParams表示接口参数的描述信息,@ApiImplicitParam表示具体的参数描述,@GetMapping表示接口的请求方式和请求路径,@RequestParam表示获取请求参数。selectUserInfoById方法是业务层的代码,根据传入的id参数查询用户信息并返回。
相关问题
通过控制层代码编写出业务层代码 @ApiOperation(value = "根据ID获取用户信息") @ApiImplicitParams(value = { @ApiImplicitParam(name = "id", value = "用户ID", dataType = "Integer", dataTypeClass = Integer.class), }) @GetMapping("/select/id") public UserInfo selectUserInfoById(@RequestParam Integer id ) throws Exception { return deviceService.selectUserInfoById(id); }
这段代码是控制层的代码,它调用了deviceService中的selectUserInfoById方法来获取用户信息。
下面是通过控制层代码编写出的业务层代码:
```java
@Service
public class DeviceService {
@Autowired
private UserInfoMapper userInfoMapper;
public UserInfo selectUserInfoById(Integer id) throws Exception {
if (id == null) {
throw new Exception("用户ID不能为空");
}
UserInfo userInfo = userInfoMapper.selectByPrimaryKey(id);
if (userInfo == null) {
throw new Exception("用户不存在");
}
return userInfo;
}
}
```
这段代码是业务层的代码,它通过调用userInfoMapper中的selectByPrimaryKey方法来获取用户信息。在方法中添加了参数校验,如果id为空或用户不存在则抛出异常。
@ApiOperation(value = "根据名称和年龄段查找用户") // 修改API文档描述 @ApiImplicitParams(value = { @ApiImplicitParam(name = "名称", value = "年龄段", dataTypeClass = String.class) }) @GetMapping ("/find") public UserInfo findDeviceInfo(@RequestParam String name,@RequestParam int age) throws Exception { return deviceService.findUserInfoByIdAndName(age, name); }通过控制层代码编写出业务层代码
根据你提供的控制层代码,我们可以编写出相应的业务层代码,如下所示:
```java
@Service
public class DeviceService {
@Autowired
private UserRepository userRepository;
public UserInfo findUserInfoByIdAndName(int age, String name) {
return userRepository.findByNameAndAge(name, age);
}
}
```
其中,`UserRepository` 是一个 DAO 层的接口,用于从数据库中获取用户信息。可以使用 Spring Data JPA 等 ORM 框架来实现该接口。在该接口中,应该定义一个 `findByNameAndAge` 方法,用于根据用户的姓名和年龄段查找用户信息。具体实现方式可以参考 ORM 框架的文档。
在业务层代码中,我们注入了 `UserRepository` 对象,并且定义了一个 `findUserInfoByIdAndName` 方法,用于调用 `UserRepository` 中的 `findByNameAndAge` 方法来获取用户信息。注意,参数列表的顺序与控制层代码中的相反,这是因为参数顺序与 API 文档描述中的顺序一致。
这样,我们就可以根据控制层代码编写出相应的业务层代码了。
阅读全文
相关推荐
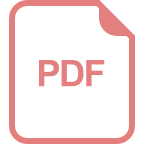
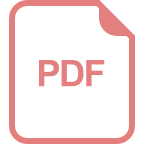

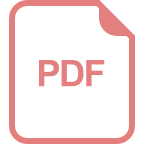
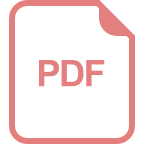
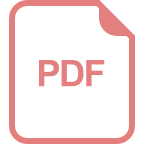
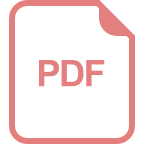
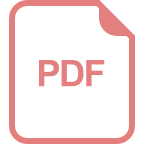
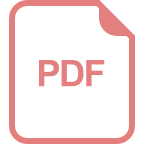
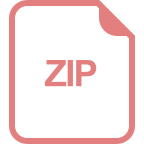
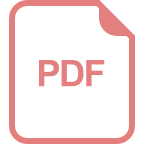
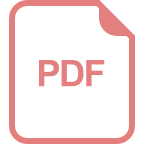
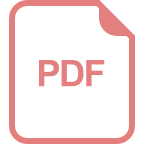


