mybatis-plus插入单条数据
时间: 2023-04-07 17:01:03 浏览: 106
可以回答这个问题。Mybatis-plus插入单条数据的方法是使用BaseMapper的insert方法,例如:
```
User user = new User();
user.setName("张三");
user.setAge(20);
userMapper.insert(user);
```
其中,userMapper是继承了BaseMapper的接口,可以直接调用insert方法插入单条数据。
相关问题
mybatis-plus批量插入数据
可以使用Mybatis-plus提供的BatchInsert方法来实现批量插入数据,示例代码如下:
```java
List<User> userList = new ArrayList<>();
// 添加多个User对象到userList中
int batchSize = 1000; // 每批次插入的数据量
int totalSize = userList.size();
int batchCount = (totalSize + batchSize - 1) / batchSize; // 计算批次数
for (int i = 0; i < batchCount; i++) {
int fromIndex = i * batchSize;
int toIndex = Math.min((i + 1) * batchSize, totalSize);
List<User> subList = userList.subList(fromIndex, toIndex);
int rows = userMapper.batchInsert(subList); // 批量插入数据
System.out.println("第" + (i + 1) + "批次插入了" + rows + "条数据");
}
```
其中,User是一个JavaBean,userMapper是Mybatis-plus自动生成的Mapper接口,batchInsert方法是自定义的批量插入方法,其实现类似于单条插入,只是需要在SQL语句中添加VALUES子句的多个值。
mybatis-plus-extension单条禁用
Mybatis-Plus Extension是一个对Mybatis Plus进行了功能增强的扩展工具包,它提供了一些方便实用的功能,如CRUD操作、定时任务等。其中,如果需要禁用单条数据的插入、更新或删除操作,通常是在执行具体业务逻辑前的一个条件判断或者配置环节。
在Mybatis-Plus中,你可以通过拦截器(Interceptor)来实现这个需求。你可以自定义一个拦截器,在`beforeInsert()`, `beforeUpdate()`, 或者 `beforeDelete()` 方法中添加判断逻辑,比如检查某个字段是否满足特定条件,如果不满足,则返回false或抛出异常,阻止操作继续执行。
例如,你可以在`beforeInsert()` 中这样做:
```java
@Around("execution(* com.example.mapper.UserMapper.insert(..))")
public Object aroundInsert(ProceedingJoinPoint joinPoint) throws Throwable {
// 获取到User实体对象
User user = (User) joinPoint.getArgs()[0];
// 判断某个字段,如 disableFlag 是否为 false
if (user.getDisableFlag()) {
throw new RuntimeException("单条数据禁止插入");
}
// 如果满足条件,正常执行插入操作
return joinPoint.proceed();
}
```
记得在启用拦截器时将其配置到全局配置文件中,并确保在对应的Mapper接口上添加相应注解,如`@Mapper interception="base"`。
阅读全文
相关推荐

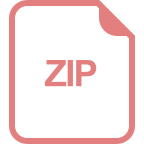
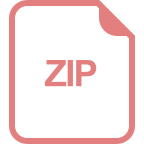
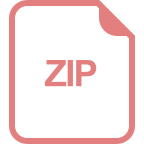
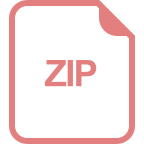
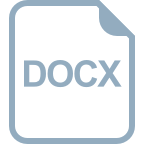
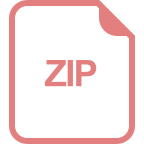
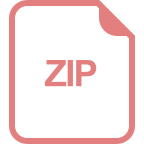
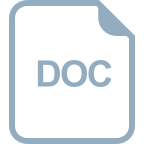
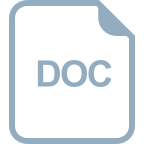
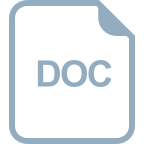
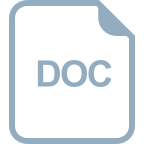
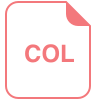