程序中定义一个有5个元素的字符串数组,使用JOptionPane类中的showInputDialog方法创建文本输入对话框,给字符串数组的5个元素赋值,
时间: 2024-02-11 10:10:04 浏览: 78
好的,以下是示例代码:
```java
import javax.swing.JOptionPane;
public class StringArrayInput {
public static void main(String[] args) {
String[] strArray = new String[5];
for (int i = 0; i < strArray.length; i++) {
String input = JOptionPane.showInputDialog("请输入第" + (i+1) + "个字符串:");
strArray[i] = input;
}
// 输出数组
for (String str : strArray) {
System.out.println(str);
}
}
}
```
运行程序后,会弹出5次文本输入对话框,让用户输入字符串,然后将这些字符串存储到字符串数组中。最后,程序会输出这个字符串数组的所有元素。
相关问题
已知可以通过javax.swing.JOptionPane.showInputDialog(“这是提示词”)函数可以在对话框中进行数据输入,返回一个字符串。要求: ①输入一个客户的年龄,如果在0~100则打印年龄,否则打印“输入年龄范围有误!” 。
可以通过以下Java代码实现这个要求:
```java
import javax.swing.JOptionPane;
public class AgeInputDemo {
public static void main(String[] args) {
String input = JOptionPane.showInputDialog("请输入客户的年龄:");
try {
int age = Integer.parseInt(input);
if (age >= 0 && age <= 100) {
System.out.println(age);
} else {
System.out.println("输入年龄范围有误!");
}
} catch (NumberFormatException e) {
System.out.println("输入格式不正确,请输入有效的整数!");
}
}
}
```
这段代码首先导入了`javax.swing.JOptionPane`类,并定义了一个`AgeInputDemo`类。在`main`方法中,使用`showInputDialog`函数弹出一个输入对话框,让用户输入年龄。然后尝试将输入的字符串转换为整数,并检查该整数是否在0到100之间。如果不在这个范围内,就打印出“输入年龄范围有误!”。如果用户输入的不是整数格式,`NumberFormatException`异常会被捕获,并提示用户输入格式不正确。
使用JOptionPane.showOptionDialog()方法,定义对话框,不需要点击,继续执行程序
以下是一个示例代码:
```
import javax.swing.*;
public class OptionDialogExample {
public static void main(String[] args) {
String[] options = {"Option 1", "Option 2", "Option 3"};
int selectedOption = JOptionPane.showOptionDialog(null, "Choose an option", "Option Dialog", JOptionPane.DEFAULT_OPTION, JOptionPane.PLAIN_MESSAGE, null, options, options[0]);
System.out.println("Selected option: " + selectedOption);
//rest of the program continues here
}
}
```
此代码将创建一个包含三个选项的对话框,用户可以选择其中一个选项。`JOptionPane.showOptionDialog()`方法将返回用户选择的选项的索引,如果用户单击对话框上的关闭按钮,则将返回`-1`。程序将继续执行,无论用户是否选择了选项。在此示例中,程序将打印用户选择的选项的索引。
阅读全文
相关推荐
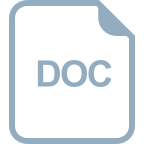
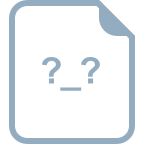
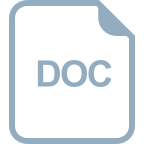
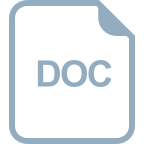










