51单片机ds1302可调时钟
时间: 2023-07-24 11:16:48 浏览: 56
DS1302是一款实时时钟芯片,可以与51单片机进行通信,实现可调时钟的功能。以下是基本的步骤:
1. 设置DS1302的时钟寄存器,包括年、月、日、时、分、秒等。
2. 设置DS1302的控制寄存器,包括时钟启动、写保护等。
3. 通过51单片机的IO口与DS1302进行通信,读取或写入寄存器。
4. 在程序中实现对时钟的调整和显示功能。
需要注意的是,DS1302的时钟精度不高,通常只能达到一分钟左右的误差。如果需要更高的精度,可以考虑使用其他实时时钟模块或芯片。
相关问题
51单片机ds1302可调时钟程序
以下是51单片机通过DS1302实现可调时钟的基本程序,供参考:
```c
#include <reg51.h>
#include <ds1302.h>
sbit SDA = P3^4;
sbit SCLK = P3^5;
sbit RST = P3^6;
void main()
{
ds1302_init(SDA, SCLK, RST); // 初始化DS1302
// 设置初始时间
ds1302_set_time(2021, 7, 1, 0, 0, 0);
while(1)
{
// 读取当前时间
unsigned char year, month, day, hour, minute, second;
ds1302_get_time(&year, &month, &day, &hour, &minute, &second);
// TODO: 在OLED屏幕或数码管上显示时间
// 按下按键进行时间调整
if(key_pressed())
{
// TODO: 在OLED屏幕或数码管上显示当前编辑的时间部分,并通过按键进行调整
// 调整完成后,通过ds1302_set_time()函数进行更新
}
}
}
```
其中,`ds1302.h`文件为DS1302驱动的头文件,包含了DS1302的初始化、读写寄存器、读写时间等函数。`key_pressed()`函数为检测按键是否按下的函数,具体实现可以根据硬件平台进行修改。其他需要实现的部分,比如时间的显示和调整,可以根据具体需求进行编写。
51单片机ds1302电子时钟
您想了解关于51单片机和ds1302电子时钟相关的内容吗?如果是的话,我可以为您提供一些基本的信息。51单片机是一种基于Intel 8051架构的单片机,常用于嵌入式系统中。DS1302是一种实时时钟芯片,具有时钟、日历和闹钟功能,可与51单片机等微控制器进行通信。通过使用51单片机和DS1302电子时钟,您可以构建一个精确可靠的时钟系统,可用于各种应用,如电子表、计时器、闹钟等。如果您有其他问题,请随时问我。
相关推荐
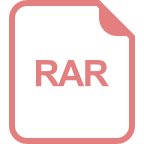
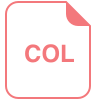
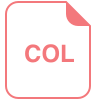
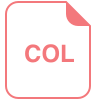









