用android实现注册界面效果
时间: 2024-05-25 20:05:46 浏览: 81
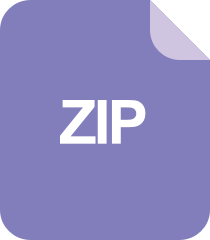
Android注册界面

由于您没有提供具体的注册界面效果,我将提供一个基本的注册界面实现方法,您可以根据自己的需求进行修改和优化。
首先,在XML布局文件中创建一个注册界面的布局。可以使用LinearLayout或RelativeLayout等布局容器来设计您的界面。
例如,以下是一个基本的注册界面布局:
```
<RelativeLayout
android:layout_width="match_parent"
android:layout_height="match_parent"
android:background="@color/white">
<TextView
android:id="@+id/textView_username"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="@string/username"
android:textColor="@color/black"
android:textSize="16sp"
android:layout_marginTop="20dp"
android:layout_marginLeft="20dp"/>
<EditText
android:id="@+id/editText_username"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:hint="@string/username_hint"
android:textColorHint="@color/grey"
android:textColor="@color/black"
android:inputType="text"
android:layout_below="@id/textView_username"
android:layout_marginTop="10dp"
android:layout_marginLeft="20dp"
android:layout_marginRight="20dp"/>
<TextView
android:id="@+id/textView_password"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="@string/password"
android:textColor="@color/black"
android:textSize="16sp"
android:layout_below="@id/editText_username"
android:layout_marginTop="20dp"
android:layout_marginLeft="20dp"/>
<EditText
android:id="@+id/editText_password"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:hint="@string/password_hint"
android:textColorHint="@color/grey"
android:textColor="@color/black"
android:inputType="textPassword"
android:layout_below="@id/textView_password"
android:layout_marginTop="10dp"
android:layout_marginLeft="20dp"
android:layout_marginRight="20dp"/>
<Button
android:id="@+id/button_register"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="@string/register"
android:background="@drawable/button_background"
android:textColor="@color/white"
android:textSize="16sp"
android:layout_below="@id/editText_password"
android:layout_marginTop="30dp"
android:layout_marginLeft="20dp"
android:layout_marginRight="20dp"/>
</RelativeLayout>
```
接下来,您需要在Java代码中实现注册功能。在您的Activity或Fragment中,创建一个方法来处理用户的注册请求。该方法应该包括以下步骤:
1. 获取用户名和密码EditText的值。
2. 验证用户名和密码是否为空或无效。
3. 发送注册请求到服务器。
4. 处理服务器响应并显示适当的消息。
以下是一个简单的注册方法示例:
```
private void registerUser() {
String username = editText_username.getText().toString().trim();
String password = editText_password.getText().toString().trim();
if(username.isEmpty()) {
editText_username.setError("Please enter username");
editText_username.requestFocus();
return;
}
if(password.isEmpty()) {
editText_password.setError("Please enter password");
editText_password.requestFocus();
return;
}
// 发送注册请求到服务器
// ...
// 处理服务器响应并显示适当的消息
// ...
}
```
最后,您需要在注册按钮的点击事件中调用该方法。例如,您可以在onCreate方法中为注册按钮添加一个点击事件监听器:
```
Button buttonRegister = findViewById(R.id.button_register);
buttonRegister.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
registerUser();
}
});
```
以上就是一个基本的注册界面实现方法。您可以根据自己的需求进行自定义和优化。
阅读全文
相关推荐




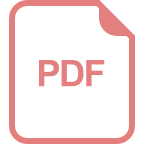
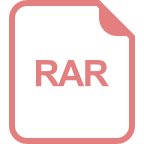
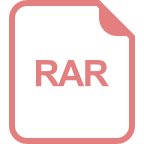
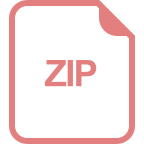
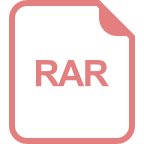
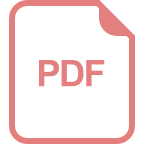
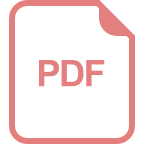
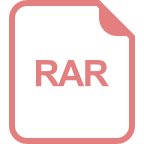
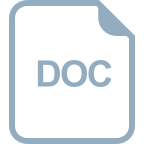
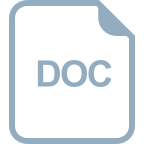

