C语言编程问题描述】编写一个程序,该程序将查找,搜索和替换文本字符串。 程序从输入中读取文本直到行尾。 然后,您在文本中搜索短语,并输入替换短语替换所有搜索的短语。 替换短语可以比搜索短语短或长。【样例输入】 This is my first line in my first text first second 【样例输出】 This is my second line in my second text
时间: 2024-01-22 22:20:53 浏览: 69
以下是使用C语言编写的程序,可以实现查找、搜索和替换文本字符串。程序首先从标准输入读取一行文本,然后用户输入要查找的短语和替换短语。程序接着在文本中搜索并替换所有出现的短语,并将结果输出到标准输出。
```c
#include <stdio.h>
#include <string.h>
#define MAX_LINE_LENGTH 1024
int main()
{
char line[MAX_LINE_LENGTH];
char search_phrase[MAX_LINE_LENGTH];
char replace_phrase[MAX_LINE_LENGTH];
int search_phrase_length, replace_phrase_length;
// 读取输入的文本
fgets(line, MAX_LINE_LENGTH, stdin);
// 读取要查找和替换的短语
printf("Enter search phrase: ");
fgets(search_phrase, MAX_LINE_LENGTH, stdin);
printf("Enter replace phrase: ");
fgets(replace_phrase, MAX_LINE_LENGTH, stdin);
// 去掉短语中的换行符
search_phrase_length = strlen(search_phrase);
replace_phrase_length = strlen(replace_phrase);
if (search_phrase[search_phrase_length - 1] == '\n') {
search_phrase[search_phrase_length - 1] = '\0';
}
if (replace_phrase[replace_phrase_length - 1] == '\n') {
replace_phrase[replace_phrase_length - 1] = '\0';
}
// 搜索并替换短语
char *pos = line;
while ((pos = strstr(pos, search_phrase)) != NULL) {
memmove(pos + replace_phrase_length, pos + search_phrase_length, strlen(pos + search_phrase_length) + 1);
memcpy(pos, replace_phrase, replace_phrase_length);
pos += replace_phrase_length;
}
// 输出结果
printf("%s", line);
return 0;
}
```
在上面的示例中,我们使用了`fgets()`函数来从标准输入读取文本和短语。`fgets()`函数可以读取一行文本,并将其存储在一个字符数组中。我们还使用了`strstr()`函数在文本中搜索短语,并将其替换为另一个短语。最后,我们使用`printf()`函数将结果输出到标准输出。
阅读全文
相关推荐
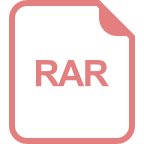


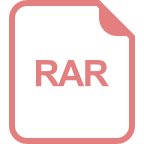
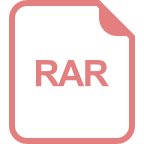
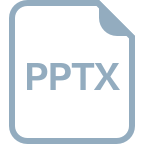
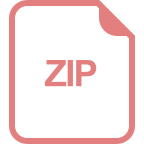
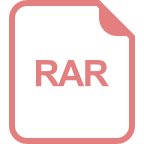
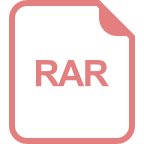
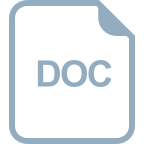
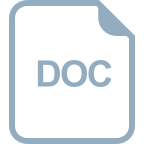
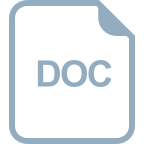
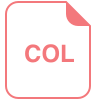
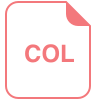
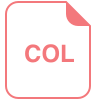
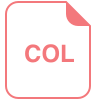