在vue2项目配置一个打包速度很快的webpack文件
时间: 2024-03-17 15:45:34 浏览: 18
要在Vue2项目中配置一个打包速度很快的Webpack文件,可以参考以下步骤:
1. 使用`vue-cli`创建一个Vue2项目:
```
vue create my-project
```
2. 安装`webpack-merge`插件,用于合并Webpack配置:
```
npm install --save-dev webpack-merge
```
3. 在项目根目录下创建一个`build`目录,并在其中创建一个名为`webpack.common.js`的文件,用于存放公共的Webpack配置:
```
const path = require('path');
const webpack = require('webpack');
const { VueLoaderPlugin } = require('vue-loader');
module.exports = {
entry: {
app: './src/main.js'
},
output: {
path: path.resolve(__dirname, '../dist'),
filename: '[name].js'
},
resolve: {
extensions: ['.js', '.vue', '.json'],
alias: {
'@': path.resolve(__dirname, '../src')
}
},
module: {
rules: [
{
test: /\.vue$/,
loader: 'vue-loader'
},
{
test: /\.js$/,
loader: 'babel-loader',
exclude: /node_modules/
},
{
test: /\.(png|jpe?g|gif|svg)(\?.*)?$/,
loader: 'url-loader',
options: {
limit: 10000,
name: 'img/[name].[hash:7].[ext]'
}
},
{
test: /\.(woff2?|eot|ttf|otf)(\?.*)?$/,
loader: 'url-loader',
options: {
limit: 10000,
name: 'fonts/[name].[hash:7].[ext]'
}
}
]
},
plugins: [
new VueLoaderPlugin(),
new webpack.optimize.ModuleConcatenationPlugin()
]
};
```
4. 在`build`目录下创建一个名为`webpack.dev.js`的文件,用于存放开发环境的Webpack配置:
```
const merge = require('webpack-merge');
const common = require('./webpack.common.js');
module.exports = merge(common, {
mode: 'development',
devtool: 'cheap-module-eval-source-map',
devServer: {
contentBase: '../dist',
hot: true
},
module: {
rules: [
{
test: /\.css$/,
use: [
'style-loader',
'css-loader'
]
},
{
test: /\.scss$/,
use: [
'style-loader',
'css-loader',
'sass-loader'
]
}
]
},
plugins: [
new webpack.HotModuleReplacementPlugin()
]
});
```
5. 在`build`目录下创建一个名为`webpack.prod.js`的文件,用于存放生产环境的Webpack配置:
```
const merge = require('webpack-merge');
const common = require('./webpack.common.js');
const { CleanWebpackPlugin } = require('clean-webpack-plugin');
const MiniCssExtractPlugin = require('mini-css-extract-plugin');
const OptimizeCSSAssetsPlugin = require('optimize-css-assets-webpack-plugin');
module.exports = merge(common, {
mode: 'production',
devtool: 'source-map',
optimization: {
minimizer: [
new OptimizeCSSAssetsPlugin()
]
},
module: {
rules: [
{
test: /\.css$/,
use: [
MiniCssExtractPlugin.loader,
'css-loader'
]
},
{
test: /\.scss$/,
use: [
MiniCssExtractPlugin.loader,
'css-loader',
'sass-loader'
]
}
]
},
plugins: [
new CleanWebpackPlugin(),
new MiniCssExtractPlugin({
filename: 'css/[name].[hash].css'
})
]
});
```
6. 修改`package.json`文件,添加两个命令用于启动开发和生产环境的Webpack:
```
"scripts": {
"serve": "webpack-dev-server --config build/webpack.dev.js",
"build": "webpack --config build/webpack.prod.js"
}
```
7. 运行以下命令启动开发环境的Webpack:
```
npm run serve
```
运行以下命令构建生产环境的Webpack:
```
npm run build
```
这样,你就可以在Vue2项目中配置一个打包速度很快的Webpack文件了。其中,我们使用了`webpack-merge`插件来合并公共配置和环境特定的配置,使用了`clean-webpack-plugin`插件来清空打包目录,在生产环境中使用了`mini-css-extract-plugin`插件来提取CSS,使用了`optimize-css-assets-webpack-plugin`插件来优化CSS。
相关推荐
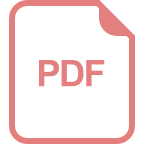
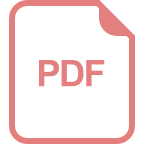
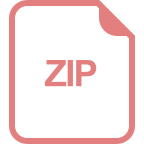
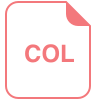
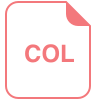
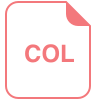
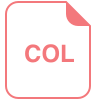
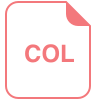









