帮我用eclipse帮我用java语言写一个小球打砖块游戏写一个小球打砖块游戏
时间: 2023-12-20 17:05:39 浏览: 91
当然可以,我可以给你提供一个简单的Java程序,它实现了小球打砖块游戏的基本功能。你可以在此基础上进行修改和完善。以下是代码:
```java
import java.awt.Color;
import java.awt.Graphics;
import java.awt.Rectangle;
import java.awt.event.KeyEvent;
import java.awt.event.KeyListener;
import java.util.ArrayList;
import java.util.List;
import javax.swing.JFrame;
import javax.swing.JPanel;
public class BallGame extends JPanel implements KeyListener {
private static final long serialVersionUID = 1L;
private int width = 800;
private int height = 600;
private List<Brick> bricks; // 砖块
private Ball ball; // 球
private Paddle paddle; // 挡板
private int score = 0; // 得分
public BallGame() {
bricks = new ArrayList<>();
for (int i = 0; i < 8; i++) {
for (int j = 0; j < 5; j++) {
Brick brick = new Brick(70 * i + 80, 20 * j + 50);
bricks.add(brick);
}
}
ball = new Ball();
paddle = new Paddle();
addKeyListener(this);
setFocusable(true);
}
public static void main(String[] args) {
BallGame game = new BallGame();
JFrame frame = new JFrame("Ball Game");
frame.setSize(game.width, game.height);
frame.setLocationRelativeTo(null);
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setContentPane(game);
frame.setVisible(true);
game.run();
}
public void run() {
while (true) {
ball.move();
paddle.move();
checkCollision();
repaint();
try {
Thread.sleep(10);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
public void checkCollision() {
// 球碰到边界
if (ball.x < 0 || ball.x > width - ball.width) {
ball.vx = -ball.vx;
}
if (ball.y < 0) {
ball.vy = -ball.vy;
}
if (ball.y > height - ball.height) {
ball.vx = 0;
ball.vy = 0;
}
// 球碰到挡板
if (ball.getBounds().intersects(paddle.getBounds())) {
ball.vy = -ball.vy;
}
// 球碰到砖块
for (Brick brick : bricks) {
if (ball.getBounds().intersects(brick.getBounds())) {
bricks.remove(brick);
score += 10;
ball.vy = -ball.vy;
break;
}
}
}
@Override
public void paint(Graphics g) {
super.paint(g);
// 画砖块
for (Brick brick : bricks) {
g.setColor(brick.color);
g.fillRect(brick.x, brick.y, brick.width, brick.height);
}
// 画球
g.setColor(ball.color);
g.fillOval(ball.x, ball.y, ball.width, ball.height);
// 画挡板
g.setColor(paddle.color);
g.fillRect(paddle.x, paddle.y, paddle.width, paddle.height);
// 画分数
g.setColor(Color.BLACK);
g.drawString("Score: " + score, 20, 30);
}
@Override
public void keyPressed(KeyEvent e) {
if (e.getKeyCode() == KeyEvent.VK_LEFT) {
paddle.vx = -5;
}
if (e.getKeyCode() == KeyEvent.VK_RIGHT) {
paddle.vx = 5;
}
}
@Override
public void keyReleased(KeyEvent e) {
if (e.getKeyCode() == KeyEvent.VK_LEFT || e.getKeyCode() == KeyEvent.VK_RIGHT) {
paddle.vx = 0;
}
}
@Override
public void keyTyped(KeyEvent e) {
}
class Brick extends Rectangle {
private static final long serialVersionUID = 1L;
public Color color;
public Brick(int x, int y) {
super(x, y, 70, 20);
color = new Color((int) (Math.random() * 0x1000000));
}
}
class Ball extends Rectangle {
private static final long serialVersionUID = 1L;
public int vx, vy;
public Color color;
public Ball() {
super(380, 550, 20, 20);
vx = 3;
vy = -3;
color = Color.RED;
}
public void move() {
x += vx;
y += vy;
}
}
class Paddle extends Rectangle {
private static final long serialVersionUID = 1L;
public int vx;
public Color color;
public Paddle() {
super(350, 570, 100, 20);
vx = 0;
color = Color.BLUE;
}
public void move() {
x += vx;
if (x < 0) {
x = 0;
}
if (x > width - width / 10) {
x = width - width / 10;
}
}
}
}
```
这个程序使用 Java Swing 组件实现了游戏界面,并使用了键盘事件监听器来控制挡板的移动。游戏中的砖块、球和挡板都是一个矩形,使用 Java 的 Rectangle 类来表示。游戏中的逻辑主要在 checkCollision 方法中实现,其中检测了球的碰撞情况,并根据不同的碰撞情况来改变球的运动状态。最后,程序使用 paint 方法来绘制游戏界面,包括砖块、球、挡板和分数。
阅读全文
相关推荐
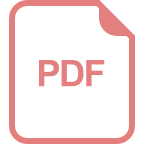
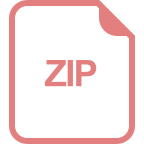
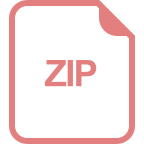















