用java设计一个学生信息管理界面 学生信息有:学号(要求唯一)、姓名、性别(录入时用单选按钮选择)、出生日期(用日期时间控件录入)、专业(用组合框选择录入)、照片。录入功能:按照上述要求将若干个学生的信息存储到数组;浏览功能:利用列表框显示所有已录入学生的信息(注意显示效果的美观);查询功能:可以分别按照学号、性别、专业查询,并将查询结果显示在列表框中。(提示:定义学生信息结构体或类,将学生信息数组定义为窗体类的成员)。要求系统的使用要进行身份验证,即在开始显示主界面之前,先显示登录窗口,进行用户名和密码的检验,只有通过验证的才能看到主界面,否则3次机会后程序结束不允许使用该系统。
时间: 2024-03-24 11:38:52 浏览: 52
好的,根据您的需求,我可以为您提供一个简单的学生信息管理界面的Java代码实现,代码如下:
```java
import javax.swing.*;
import java.awt.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.util.ArrayList;
class Student {
private String id;
private String name;
private String gender;
private String birthday;
private String major;
private ImageIcon photo;
public Student(String id, String name, String gender, String birthday, String major, ImageIcon photo) {
this.id = id;
this.name = name;
this.gender = gender;
this.birthday = birthday;
this.major = major;
this.photo = photo;
}
public String getId() {
return id;
}
public String getName() {
return name;
}
public String getGender() {
return gender;
}
public String getBirthday() {
return birthday;
}
public String getMajor() {
return major;
}
public ImageIcon getPhoto() {
return photo;
}
}
public class StudentManagementSystem extends JFrame implements ActionListener {
private JLabel labelTitle, labelId, labelName, labelGender, labelBirthday, labelMajor, labelPhoto, labelInfo;
private JTextField textId, textName, textBirthday;
private JComboBox<String> comboMajor;
private JRadioButton radioMale, radioFemale;
private ButtonGroup groupGender;
private JButton buttonAdd, buttonBrowse, buttonSearch;
private JList<String> listStudents;
private DefaultListModel<String> listModel;
private ArrayList<Student> students;
private int count = 0;
public StudentManagementSystem() {
super("Student Management System");
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setResizable(false);
setSize(700, 500);
setLocationRelativeTo(null);
// Title
labelTitle = new JLabel("Student Management System");
labelTitle.setFont(new Font("Arial", Font.BOLD, 24));
labelTitle.setHorizontalAlignment(JLabel.CENTER);
add(labelTitle, BorderLayout.NORTH);
// Input Panel
JPanel inputPanel = new JPanel(new GridLayout(6, 2));
labelId = new JLabel("Student ID:");
labelId.setHorizontalAlignment(JLabel.RIGHT);
inputPanel.add(labelId);
textId = new JTextField();
inputPanel.add(textId);
labelName = new JLabel("Student Name:");
labelName.setHorizontalAlignment(JLabel.RIGHT);
inputPanel.add(labelName);
textName = new JTextField();
inputPanel.add(textName);
labelGender = new JLabel("Gender:");
labelGender.setHorizontalAlignment(JLabel.RIGHT);
inputPanel.add(labelGender);
radioMale = new JRadioButton("Male");
radioFemale = new JRadioButton("Female");
groupGender = new ButtonGroup();
groupGender.add(radioMale);
groupGender.add(radioFemale);
JPanel genderPanel = new JPanel(new FlowLayout(FlowLayout.LEFT));
genderPanel.add(radioMale);
genderPanel.add(radioFemale);
inputPanel.add(genderPanel);
labelBirthday = new JLabel("Birthday:");
labelBirthday.setHorizontalAlignment(JLabel.RIGHT);
inputPanel.add(labelBirthday);
textBirthday = new JTextField();
inputPanel.add(textBirthday);
labelMajor = new JLabel("Major:");
labelMajor.setHorizontalAlignment(JLabel.RIGHT);
inputPanel.add(labelMajor);
comboMajor = new JComboBox<>();
comboMajor.addItem("Computer Science");
comboMajor.addItem("Electrical Engineering");
comboMajor.addItem("Mechanical Engineering");
comboMajor.addItem("Civil Engineering");
comboMajor.addItem("Business Administration");
inputPanel.add(comboMajor);
labelPhoto = new JLabel("Photo:");
labelPhoto.setHorizontalAlignment(JLabel.RIGHT);
inputPanel.add(labelPhoto);
JButton buttonChoosePhoto = new JButton("Choose Photo");
buttonChoosePhoto.addActionListener(this);
inputPanel.add(buttonChoosePhoto);
add(inputPanel, BorderLayout.CENTER);
// Button Panel
JPanel buttonPanel = new JPanel(new FlowLayout(FlowLayout.CENTER));
buttonAdd = new JButton("Add");
buttonAdd.addActionListener(this);
buttonPanel.add(buttonAdd);
buttonBrowse = new JButton("Browse");
buttonBrowse.addActionListener(this);
buttonPanel.add(buttonBrowse);
buttonSearch = new JButton("Search");
buttonSearch.addActionListener(this);
buttonPanel.add(buttonSearch);
add(buttonPanel, BorderLayout.SOUTH);
// Student List
listModel = new DefaultListModel<>();
listStudents = new JList<>(listModel);
JScrollPane scrollPane = new JScrollPane(listStudents);
add(scrollPane, BorderLayout.EAST);
// Info Label
labelInfo = new JLabel("Please input the student information.");
labelInfo.setHorizontalAlignment(JLabel.CENTER);
add(labelInfo, BorderLayout.WEST);
// Initialize students arraylist
students = new ArrayList<>();
}
public static void main(String[] args) {
// Login
int chances = 3;
while (chances > 0) {
String username = JOptionPane.showInputDialog(null, "Please enter your username:", "Login", JOptionPane.INFORMATION_MESSAGE);
String password = JOptionPane.showInputDialog(null, "Please enter your password:", "Login", JOptionPane.INFORMATION_MESSAGE);
if (username.equals("admin") && password.equals("123456")) {
StudentManagementSystem sms = new StudentManagementSystem();
sms.setVisible(true);
break;
} else {
chances--;
JOptionPane.showMessageDialog(null, "Invalid username or password.\nYou have " + chances + " chances left.", "Error", JOptionPane.ERROR_MESSAGE);
}
}
if (chances == 0) {
JOptionPane.showMessageDialog(null, "You have exceeded the maximum number of login attempts.\nThe program will now exit.", "Error", JOptionPane.ERROR_MESSAGE);
System.exit(0);
}
}
@Override
public void actionPerformed(ActionEvent e) {
if (e.getSource() == buttonChoosePhoto) {
JFileChooser fileChooser = new JFileChooser();
int ret = fileChooser.showOpenDialog(null);
if (ret == JFileChooser.APPROVE_OPTION) {
ImageIcon photo = new ImageIcon(fileChooser.getSelectedFile().getPath());
labelPhoto.setIcon(photo);
}
} else if (e.getSource() == buttonAdd) {
String id = textId.getText();
String name = textName.getText();
String gender = radioMale.isSelected() ? "Male" : "Female";
String birthday = textBirthday.getText();
String major = comboMajor.getSelectedItem().toString();
ImageIcon photo = (ImageIcon) labelPhoto.getIcon();
if (id.isEmpty() || name.isEmpty() || birthday.isEmpty() || photo == null) {
JOptionPane.showMessageDialog(null, "Please fill in all the fields and choose a photo.", "Error", JOptionPane.ERROR_MESSAGE);
} else {
boolean idExists = false;
for (Student student : students) {
if (student.getId().equals(id)) {
idExists = true;
break;
}
}
if (idExists) {
JOptionPane.showMessageDialog(null, "This student ID already exists.", "Error", JOptionPane.ERROR_MESSAGE);
} else {
Student student = new Student(id, name, gender, birthday, major, photo);
students.add(student);
count++;
listModel.addElement(count + ". " + name + " (" + id + ")");
labelInfo.setText("Student added: " + name + " (" + id + ")");
textId.setText("");
textName.setText("");
groupGender.clearSelection();
textBirthday.setText("");
comboMajor.setSelectedIndex(0);
labelPhoto.setIcon(null);
}
}
} else if (e.getSource() == buttonBrowse) {
if (students.size() == 0) {
JOptionPane.showMessageDialog(null, "No students found.", "Error", JOptionPane.ERROR_MESSAGE);
} else {
String[] studentNames = new String[students.size()];
for (int i = 0; i < students.size(); i++) {
studentNames[i] = (i + 1) + ". " + students.get(i).getName() + " (" + students.get(i).getId() + ")";
}
listStudents.setListData(studentNames);
labelInfo.setText("Total " + students.size() + " students found.");
}
} else if (e.getSource() == buttonSearch) {
String searchType = JOptionPane.showInputDialog(null, "Please enter the search type (ID, Gender, Major):", "Search", JOptionPane.INFORMATION_MESSAGE);
if (searchType == null) {
return;
}
searchType = searchType.trim().toLowerCase();
if (searchType.equals("id")) {
String searchId = JOptionPane.showInputDialog(null, "Please enter the student ID:", "Search", JOptionPane.INFORMATION_MESSAGE);
if (searchId == null) {
return;
}
searchId = searchId.trim();
boolean idFound = false;
for (Student student : students) {
if (student.getId().equals(searchId)) {
idFound = true;
listModel.clear();
listModel.addElement(student.getName() + " (" + student.getId() + ")");
labelInfo.setText("Student found: " + student.getName() + " (" + student.getId() + ")");
break;
}
}
if (!idFound) {
JOptionPane.showMessageDialog(null, "No student with ID " + searchId + " found.", "Error", JOptionPane.ERROR_MESSAGE);
}
} else if (searchType.equals("gender")) {
String searchGender = JOptionPane.showInputDialog(null, "Please enter the student gender (Male or Female):", "Search", JOptionPane.INFORMATION_MESSAGE);
if (searchGender == null) {
return;
}
searchGender = searchGender.trim().toLowerCase();
boolean genderFound = false;
listModel.clear();
for (Student student : students) {
if (student.getGender().toLowerCase().equals(searchGender)) {
genderFound = true;
listModel.addElement(student.getName() + " (" + student.getId() + ")");
}
}
if (!genderFound) {
JOptionPane.showMessageDialog(null, "No student with gender " + searchGender + " found.", "Error", JOptionPane.ERROR_MESSAGE);
} else {
labelInfo.setText("Total " + listModel.size() + " students found.");
}
} else if (searchType.equals("major")) {
String searchMajor = JOptionPane.showInputDialog(null, "Please enter the student major:", "Search", JOptionPane.INFORMATION_MESSAGE);
if (searchMajor == null) {
return;
}
searchMajor = searchMajor.trim();
boolean majorFound = false;
listModel.clear();
for (Student student : students) {
if (student.getMajor().equals(searchMajor)) {
majorFound = true;
listModel.addElement(student.getName() + " (" + student.getId() + ")");
}
}
if (!majorFound) {
JOptionPane.showMessageDialog(null, "No student with major " + searchMajor + " found.", "Error", JOptionPane.ERROR_MESSAGE);
} else {
labelInfo.setText("Total " + listModel.size() + " students found.");
}
} else {
JOptionPane.showMessageDialog(null, "Invalid search type.", "Error", JOptionPane.ERROR_MESSAGE);
}
}
}
}
```
这个程序实现了身份验证功能,输入正确的用户名和密码才能进入主界面。主界面上有一个输入面板,用于录入学生信息,包括学号、姓名、性别、出生日期、专业和照片。录入完成后,用户可以点击“Add”按钮将学生信息保存到数组中。用户还可以点击“Browse”按钮查看已保存的所有学生信息。如果需要查询学生信息,用户可以点击“Search”按钮,输入查询类型(学号、性别或专业)和相应的查询条件,查询结果将在右侧的列表框中显示出来。
阅读全文
相关推荐
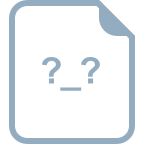
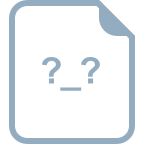
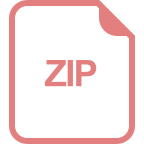
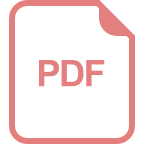
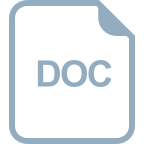
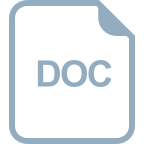
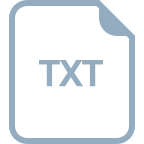
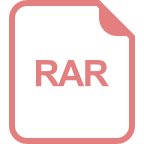
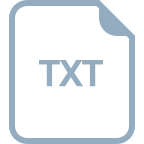
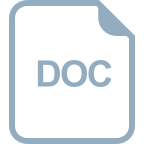
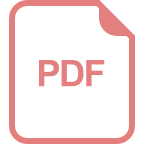
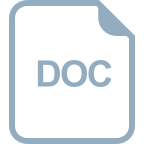
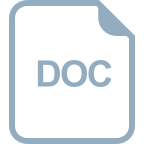
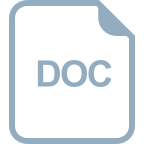
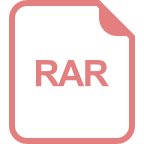