用Java生成一个可以在线聊天的小程序,并讲解具体实现步骤
时间: 2024-02-01 19:16:21 浏览: 137
生成一个可以在线聊天的小程序,需要用到Java Servlet和WebSocket技术。具体实现步骤如下:
1. 创建一个Java Web工程,导入WebSocket相关jar包,如:javax.websocket-api、tyrus-server、tyrus-client等。
2. 在web.xml文件中配置WebSocket的Servlet,如下所示:
```
<servlet>
<servlet-name>ChatServlet</servlet-name>
<servlet-class>org.glassfish.tyrus.servlet.TyrusServlet</servlet-class>
<init-param>
<param-name>org.glassfish.tyrus.server.Server</param-name>
<param-value>com.example.ChatServer</param-value>
</init-param>
<load-on-startup>1</load-on-startup>
</servlet>
<servlet-mapping>
<servlet-name>ChatServlet</servlet-name>
<url-pattern>/chat/*</url-pattern>
</servlet-mapping>
```
3. 创建一个实现了javax.websocket.Endpoint接口的类,如下所示:
```
@ServerEndpoint(value = "/chat/{username}")
public class ChatEndpoint {
private static final Set<ChatEndpoint> chatEndpoints = new CopyOnWriteArraySet<>();
private static final Map<String, String> users = new ConcurrentHashMap<>();
private Session session;
private String username;
@OnOpen
public void onOpen(Session session, @PathParam("username") String username) throws IOException {
this.session = session;
this.username = username;
chatEndpoints.add(this);
users.put(session.getId(), username);
String message = String.format("%s joined the chat room.", username);
broadcast(message);
}
@OnMessage
public void onMessage(String message, Session session) throws IOException {
String username = users.get(session.getId());
String formattedMessage = String.format("%s: %s", username, message);
broadcast(formattedMessage);
}
@OnClose
public void onClose(Session session) throws IOException {
chatEndpoints.remove(this);
String username = users.remove(session.getId());
String message = String.format("%s left the chat room.", username);
broadcast(message);
}
@OnError
public void onError(Session session, Throwable throwable) {
// Do error handling here
}
private static void broadcast(String message) throws IOException {
for (ChatEndpoint endpoint : chatEndpoints) {
synchronized (endpoint) {
endpoint.session.getBasicRemote().sendText(message);
}
}
}
}
```
4. 创建一个WebSocket服务器,如下所示:
```
public class ChatServer {
public static void main(String[] args) {
Server server = new Server("localhost", 8025, "/chat", null, ChatEndpoint.class);
try {
server.start();
System.in.read();
} catch (Exception e) {
throw new RuntimeException(e);
} finally {
server.stop();
}
}
}
```
5. 创建一个JSP页面,用于显示聊天内容和发送消息,如下所示:
```
<%@ page contentType="text/html;charset=UTF-8" language="java" %>
<%@ page import="java.util.*" %>
<%@ page import="javax.websocket.*" %>
<%@ page import="java.net.URI" %>
<html>
<head>
<title>Chat Room</title>
<script type="text/javascript">
var socket;
function connect() {
var username = document.getElementById("username").value;
var url = "ws://" + document.location.host + document.location.pathname + "chat/" + username;
socket = new WebSocket(url);
socket.onopen = function(event) {
updateStatus("Connected to WebSocket server.");
}
socket.onmessage = function(event) {
var message = event.data;
var div = document.createElement("div");
div.innerHTML = message;
document.getElementById("chat").appendChild(div);
}
socket.onclose = function(event) {
updateStatus("Disconnected from WebSocket server.");
}
}
function disconnect() {
socket.close();
}
function sendMessage() {
var message = document.getElementById("message").value;
socket.send(message);
}
function updateStatus(status) {
document.getElementById("status").innerHTML = status;
}
</script>
</head>
<body onload="connect()">
<h1>Chat Room</h1>
<div id="status"></div>
<div id="chat"></div>
<input type="text" id="username" placeholder="Enter your username">
<input type="text" id="message" placeholder="Enter your message">
<button onclick="sendMessage()">Send</button>
<button onclick="disconnect()">Disconnect</button>
</body>
</html>
```
6. 运行WebSocket服务器,并在浏览器中访问JSP页面,即可进入在线聊天室。
以上就是用Java生成一个可以在线聊天的小程序的具体实现步骤。
阅读全文
相关推荐






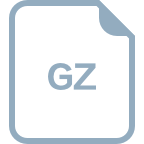
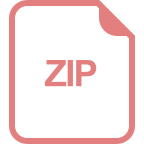










