使用imgaug库对labelimg标注后的数据进行增强
时间: 2023-08-05 18:46:09 浏览: 45
好的,我可以回答这个问题。首先,确保你已经安装了imgaug库。然后,你可以按照以下步骤对labelimg标注后的数据进行增强:
1. 读取labelimg标注后的数据,包括图像和标注信息。
2. 使用imgaug库的各种增强技术对图像进行增强,例如旋转、缩放、平移、镜像等。
3. 对标注信息进行相应的调整,确保它们与增强后的图像一致。
4. 将增强后的图像和标注信息保存到新的文件中。
下面是一个示例代码,演示了如何使用imgaug库对labelimg标注后的数据进行增强:
```python
import imgaug.augmenters as iaa
import cv2
import xml.etree.ElementTree as ET
import os
# 读取标注文件
def parse_xml_annotation(xml_file):
tree = ET.parse(xml_file)
root = tree.getroot()
boxes = []
for obj in root.findall('object'):
name = obj.find('name').text
xmin = int(obj.find('bndbox/xmin').text)
ymin = int(obj.find('bndbox/ymin').text)
xmax = int(obj.find('bndbox/xmax').text)
ymax = int(obj.find('bndbox/ymax').text)
boxes.append([xmin, ymin, xmax, ymax, name])
return boxes
# 定义增强器
seq = iaa.Sequential([
iaa.Fliplr(0.5), # 随机水平翻转
iaa.Affine(rotate=(-10, 10)), # 随机旋转
iaa.Affine(scale=(0.5, 1.5)), # 随机缩放
iaa.Affine(translate_percent={"x": (-0.2, 0.2), "y": (-0.2, 0.2)}) # 随机平移
])
# 处理每个图像
img_dir = "path/to/images"
label_dir = "path/to/labels"
for img_file in os.listdir(img_dir):
img_path = os.path.join(img_dir, img_file)
label_file = os.path.splitext(img_file)[0] + ".xml"
label_path = os.path.join(label_dir, label_file)
# 读取图像和标注信息
img = cv2.imread(img_path)
boxes = parse_xml_annotation(label_path)
# 执行增强操作
img_aug, boxes_aug = seq(image=img, bounding_boxes=boxes)
# 保存增强后的图像和标注信息
cv2.imwrite("path/to/augmented/images/{}.jpg".format(os.path.splitext(img_file)[0]), img_aug)
with open("path/to/augmented/labels/{}.xml".format(os.path.splitext(img_file)[0]), "w") as f:
f.write("<annotation>\n")
f.write(" <filename>{}</filename>\n".format(os.path.splitext(img_file)[0] + ".jpg"))
f.write(" <size>\n")
f.write(" <width>{}</width>\n".format(img_aug.shape[1]))
f.write(" <height>{}</height>\n".format(img_aug.shape[0]))
f.write(" </size>\n")
for box in boxes_aug:
f.write(" <object>\n")
f.write(" <name>{}</name>\n".format(box[4]))
f.write(" <bndbox>\n")
f.write(" <xmin>{}</xmin>\n".format(box[0]))
f.write(" <ymin>{}</ymin>\n".format(box[1]))
f.write(" <xmax>{}</xmax>\n".format(box[2]))
f.write(" <ymax>{}</ymax>\n".format(box[3]))
f.write(" </bndbox>\n")
f.write(" </object>\n")
f.write("</annotation>\n")
```
这段代码会对指定目录下的所有图像进行增强,并将增强后的图像和标注信息保存到新的目录中。你可以根据自己的需求修改这段代码。
相关推荐
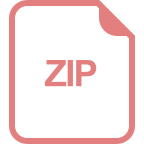
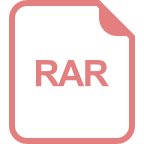
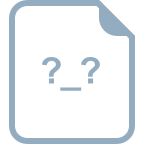
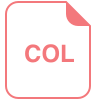
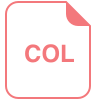
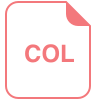
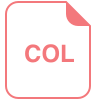
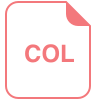









