opencv不规则图像轮廓匹配并截取c++代码实现
时间: 2023-11-13 11:05:50 浏览: 205
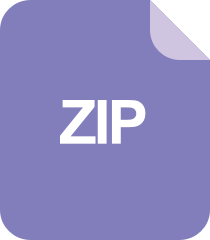
基于Opencv + ORB特征匹配算法的图像拼接c++源码(代码注释).zip

以下是使用 OpenCV 在 C++ 中实现不规则图像轮廓匹配并截取的示例代码:
```cpp
#include <opencv2/opencv.hpp>
#include <iostream>
using namespace cv;
using namespace std;
int main(int argc, char** argv)
{
// 读取原图和模板图
Mat src = imread("src.jpg", IMREAD_COLOR);
Mat tpl = imread("tpl.jpg", IMREAD_COLOR);
// 灰度化
Mat src_gray, tpl_gray;
cvtColor(src, src_gray, COLOR_BGR2GRAY);
cvtColor(tpl, tpl_gray, COLOR_BGR2GRAY);
// 二值化
Mat src_bin, tpl_bin;
threshold(src_gray, src_bin, 0, 255, THRESH_BINARY);
threshold(tpl_gray, tpl_bin, 0, 255, THRESH_BINARY);
// 寻找轮廓
vector<vector<Point>> src_contours, tpl_contours;
vector<Vec4i> src_hierarchy, tpl_hierarchy;
findContours(src_bin, src_contours, src_hierarchy, RETR_TREE, CHAIN_APPROX_SIMPLE);
findContours(tpl_bin, tpl_contours, tpl_hierarchy, RETR_TREE, CHAIN_APPROX_SIMPLE);
// 找到最佳匹配的轮廓
double max_score = 0;
int max_idx = 0;
for (size_t i = 0; i < tpl_contours.size(); i++) {
double score = matchShapes(tpl_contours[i], src_contours[0], CONTOURS_MATCH_I1, 0);
if (score > max_score) {
max_score = score;
max_idx = i;
}
}
// 截取匹配到的轮廓区域
Rect roi = boundingRect(tpl_contours[max_idx]);
Mat result = src(roi);
// 显示结果
imshow("src", src);
imshow("tpl", tpl);
imshow("result", result);
waitKey(0);
return 0;
}
```
这段代码首先读取原图和模板图,并将它们灰度化和二值化。然后使用 `findContours` 函数寻找二值化后的图像中的轮廓。接下来,对于模板图中的每个轮廓,使用 `matchShapes` 函数计算与原图中轮廓的相似度,并找到最佳匹配的轮廓。最后,使用 `boundingRect` 函数找到匹配到的轮廓的边界框,并截取原图中的对应区域作为最终结果。
阅读全文
相关推荐
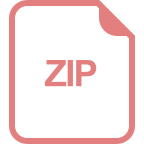

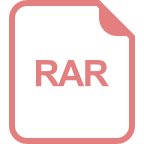
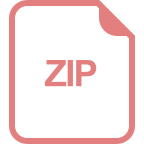
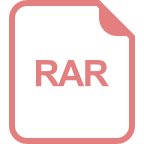
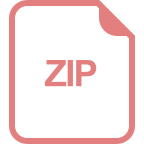
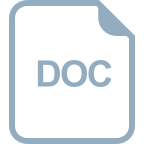
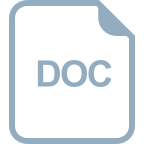
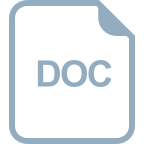
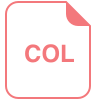
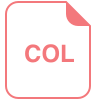

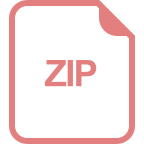