python 画折线图两个
时间: 2023-09-01 17:07:15 浏览: 39
变量如何实现?
可以使用matplotlib库中的plot()函数来实现。在plot()函数中,第一个参数为横坐标数组,第二个参数为纵坐标数组,这两个数组的长度必须相等。示例代码如下:
```python
import matplotlib.pyplot as plt
# 横坐标数组
x = [1, 2, 3, 4, 5]
# 纵坐标数组1
y1 = [7, 11, 15, 18, 20]
# 纵坐标数组2
y2 = [2, 5, 9, 12, 15]
# 绘制折线图
plt.plot(x, y1)
plt.plot(x, y2)
# 设置图表标题和坐标轴标签
plt.title("Line Chart")
plt.xlabel("X-axis")
plt.ylabel("Y-axis")
# 显示图表
plt.show()
```
以上代码将绘制两条折线,一条为纵坐标数组y1,一条为纵坐标数组y2,横坐标数组x为公共的横坐标。可以根据需要修改横、纵坐标数组中的数值,以及图表标题、坐标轴标签等参数。
相关问题
python画折线图
### 回答1:
要使用Python绘制折线图,可以使用Matplotlib库。以下是一个基本的示例代码:
```python
import matplotlib.pyplot as plt
# 数据
x = [1, 2, 3, 4, 5]
y = [1, 4, 9, 16, 25]
# 绘制折线图
plt.plot(x, y)
# 添加标题和标签
plt.title("折线图示例")
plt.xlabel("X轴")
plt.ylabel("Y轴")
# 显示图形
plt.show()
```
在这个例子中,我们定义了x和y两个列表作为数据,并使用plot函数绘制折线图。然后我们添加了标题和标签,最后使用show函数显示图形。你可以根据自己的需要修改数据和标签。
如果你需要更多定制化的功能,可以参考Matplotlib的官方文档。
### 回答2:
Python 是一种强大的编程语言,可以用来进行数据可视化,并且支持绘制折线图。要使用 Python 绘制折线图,我们可以使用 matplotlib 这个常用的数据可视化库。
首先,需要在计算机上安装 matplotlib 和 numpy 这两个库。可以使用 pip 命令来安装它们:
```
pip install matplotlib
pip install numpy
```
安装完成后,我们需要导入这两个库:
```python
import matplotlib.pyplot as plt
import numpy as np
```
接下来,我们需要准备一组数据来绘制折线图。假设我们有 x 和 y 两个列表,分别表示横轴和纵轴的数据。可以使用 numpy 的 arange 方法来生成一组连续的数字作为 x 值,然后再手动指定 y 值。
```python
x = np.arange(0, 10, 0.1)
y = [np.sin(i) for i in x]
```
使用这两组数据,我们可以调用 matplotlib 的 plot 方法来绘制折线图:
```python
plt.plot(x, y)
```
最后,我们可以设置折线图的标题、标签和样式,然后调用 show 方法展示图像:
```python
plt.title("折线图示例")
plt.xlabel("时间")
plt.ylabel("数值")
plt.grid(True)
plt.show()
```
以上就是使用 Python 绘制折线图的基本流程。当然,还有很多高级用法和自定义选项可以探索,比如添加多条折线、修改线条颜色和样式等等。希望这个简单的示例对你有所帮助!
### 回答3:
Python是一种广泛应用于数据可视化的编程语言,可以使用多种库和工具来制作折线图。以下是一种基本的方法:
首先,我们需要导入相关的库:
```python
import matplotlib.pyplot as plt
```
接下来,我们准备数据。假设我们有两个列表`x`和`y`,分别存储了折线图中的横坐标和纵坐标的数值。例如:
```python
x = [1, 2, 3, 4, 5]
y = [10, 15, 7, 12, 9]
```
然后,我们使用`plt.plot()`函数来绘制折线图:
```python
plt.plot(x, y)
```
接下来,我们可以对折线图进行一些自定义的设置,例如添加标题、横坐标和纵坐标标签等:
```python
plt.title("折线图示例")
plt.xlabel("横坐标")
plt.ylabel("纵坐标")
```
最后,我们使用`plt.show()`函数来显示折线图:
```python
plt.show()
```
这样,我们就完成了使用Python绘制折线图的过程。当然,除了基本的折线图外,还可以通过使用其他函数和参数来添加图例、调整线条样式、设置坐标轴范围等,以实现更丰富的可视化效果。
python画折线图显示两条折线
实现这个需求可以使用Python中的matplotlib库。下面是一个简单的示例代码,可以画出两条折线图:
```
import matplotlib.pyplot as plt
# 定义数据
x = [1, 2, 3, 4, 5]
y1 = [5, 4, 3, 2, 1]
y2 = [1, 2, 3, 4, 5]
# 绘制折线图
plt.plot(x, y1, label='Line 1')
plt.plot(x, y2, label='Line 2')
# 添加图例、标题等
plt.legend()
plt.title('Two lines')
plt.xlabel('X-axis')
plt.ylabel('Y-axis')
# 显示图像
plt.show()
```
在这个示例中,我们首先定义了两个列表数据,分别对应两条折线的 x 坐标和 y 坐标。然后使用 plt.plot() 方法绘制出每条折线。其中,label 参数用于给每条折线添加一个标签,方便后面添加图例。最后,我们可以使用 plt.legend()、plt.title()、plt.xlabel()、plt.ylabel() 等方法添加图例、标题和坐标轴标签,最终使用 plt.show() 显示图像。
如果你需要更详细的教程和示例,可以参考Matplotlib官方文档:https://matplotlib.org/stable/tutorials/introductory/pyplot.html。
相关推荐
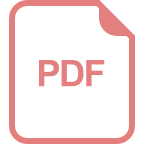
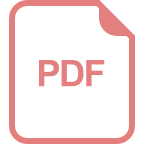
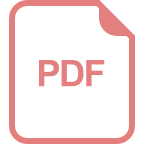












