Java8实现:利用Java-diff-utils实现自动化对比两个文件夹下的同名文件,将文件夹中是否存在,并将差异文件中的差异对象输出到result文件中。要求减少嵌套循环
时间: 2024-02-15 07:05:39 浏览: 210
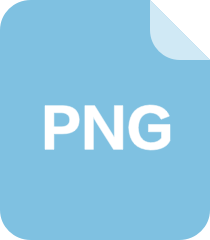
文件对比工具,用于不同的文件和文件夹,特别用于不同版本代码比较
好的,我可以帮你解答这个问题。
首先,你需要引入 Java-diff-utils 库。你可以使用 Maven 来管理依赖,添加以下代码到 pom.xml 文件中:
```xml
<dependency>
<groupId>com.googlecode.java-diff-utils</groupId>
<artifactId>diffutils</artifactId>
<version>1.3.0</version>
</dependency>
```
接下来,你可以写一个递归函数来遍历文件夹,对比同名文件的差异,并将差异对象输出到 result 文件中。以下是一个示例代码:
```java
import difflib.DiffUtils;
import difflib.Patch;
import java.io.*;
import java.util.List;
public class FolderComparator {
public static void main(String[] args) throws IOException {
String folder1Path = "path/to/folder1";
String folder2Path = "path/to/folder2";
String resultFilePath = "path/to/result.txt";
File folder1 = new File(folder1Path);
File folder2 = new File(folder2Path);
File[] files1 = folder1.listFiles();
for (File file1 : files1) {
String fileName = file1.getName();
File file2 = new File(folder2Path + "/" + fileName);
if (file2.exists()) {
if (!file1.isDirectory() && !file2.isDirectory()) {
List<String> lines1 = readLinesFromFile(file1);
List<String> lines2 = readLinesFromFile(file2);
Patch patch = DiffUtils.diff(lines1, lines2);
if (!patch.getDeltas().isEmpty()) {
writePatchToFile(fileName, patch, resultFilePath);
}
} else {
main(new String[]{file1.getAbsolutePath(), file2.getAbsolutePath(), resultFilePath});
}
}
}
}
private static List<String> readLinesFromFile(File file) throws IOException {
BufferedReader reader = new BufferedReader(new FileReader(file));
List<String> lines = new LinkedList<>();
String line;
while ((line = reader.readLine()) != null) {
lines.add(line);
}
reader.close();
return lines;
}
private static void writePatchToFile(String fileName, Patch patch, String resultFilePath) throws IOException {
BufferedWriter writer = new BufferedWriter(new FileWriter(resultFilePath, true));
writer.write("Changes in file " + fileName + ":\n");
for (Object delta : patch.getDeltas()) {
writer.write(delta.toString() + "\n");
}
writer.write("\n");
writer.close();
}
}
```
这段代码会比较两个文件夹中同名的文件,将差异对象输出到指定文件中。注意,为了减少嵌套循环,我使用了递归函数来遍历文件夹。如果文件夹中有子文件夹,递归函数会进入子文件夹中继续遍历。
希望这个答案能够帮到你,如果你有任何疑问或需要进一步的帮助,请随时提问。
阅读全文
相关推荐
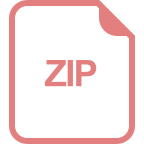
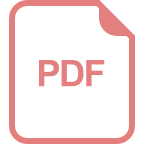








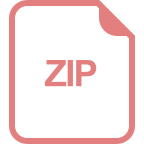
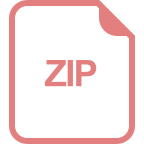
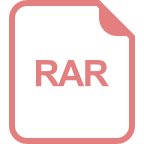
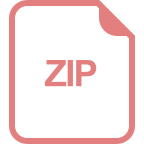
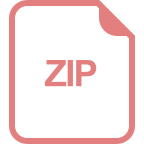
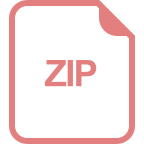
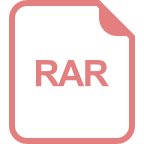