python tk 仿网易云界面
时间: 2023-07-21 12:44:06 浏览: 87
以下是一个简单的实现过程:
1. 导入必要的模块
```python
import tkinter as tk
from tkinter import ttk
from tkinter import messagebox
from PIL import Image, ImageTk
```
2. 创建主窗口和控制面板
```python
root = tk.Tk()
root.title("网易云音乐")
root.geometry("800x600")
# 创建控制面板
control_panel = tk.Frame(root, bg="#f2f2f2")
control_panel.place(x=0, y=0, relwidth=1, relheight=0.1)
# 创建主界面
main_frame = tk.Frame(root, bg="#f2f2f2")
main_frame.place(x=0, y=60, relwidth=1, relheight=0.9)
```
3. 加载图标并创建标签
```python
# 加载图标
img_logo = ImageTk.PhotoImage(Image.open("logo.png"))
# 创建标签
logo_label = tk.Label(control_panel, image=img_logo)
logo_label.pack(side="left", padx=10)
title_label = tk.Label(control_panel, text="网易云音乐", font=("微软雅黑", 20), bg="#f2f2f2")
title_label.pack(side="left", padx=10)
search_entry = tk.Entry(control_panel, width=50, font=("微软雅黑", 12))
search_entry.pack(side="left", padx=10)
search_button = tk.Button(control_panel, text="搜索", font=("微软雅黑", 12))
search_button.pack(side="left", padx=10)
```
4. 创建音乐列表
```python
# 创建音乐列表
music_list = ttk.Treeview(main_frame, columns=("title", "artist", "album", "time"), show="headings")
music_list.column("title", width=200)
music_list.column("artist", width=150)
music_list.column("album", width=100)
music_list.column("time", width=50)
music_list.heading("title", text="音乐标题")
music_list.heading("artist", text="歌手")
music_list.heading("album", text="专辑")
music_list.heading("time", text="时长")
music_list.pack(side="left", fill="both", expand=True)
```
5. 创建音乐详情面板
```python
# 创建音乐详情面板
detail_panel = tk.Frame(main_frame, bg="white")
detail_panel.place(x=400, y=0, relwidth=0.6, relheight=1)
detail_title_label = tk.Label(detail_panel, text="音乐标题", font=("微软雅黑", 20), bg="white")
detail_title_label.pack(pady=20)
detail_artist_label = tk.Label(detail_panel, text="歌手:", font=("微软雅黑", 14), bg="white")
detail_artist_label.pack(pady=10)
detail_album_label = tk.Label(detail_panel, text="专辑:", font=("微软雅黑", 14), bg="white")
detail_album_label.pack(pady=10)
detail_lyric_label = tk.Label(detail_panel, text="歌词:", font=("微软雅黑", 14), bg="white")
detail_lyric_label.pack(pady=10)
detail_lyric_text = tk.Text(detail_panel, font=("微软雅黑", 12), bg="white", width=50, height=10)
detail_lyric_text.pack(pady=10)
```
6. 定义搜索函数
```python
# 定义搜索函数
def search():
keyword = search_entry.get()
if keyword.strip() == "":
messagebox.showwarning("提示", "请输入搜索关键字!")
return
music_list.delete(*music_list.get_children()) # 清空列表
# TODO: 根据搜索关键字获取音乐列表,并添加到音乐列表中
```
7. 绑定搜索按钮事件
```python
# 绑定搜索按钮事件
search_button["command"] = search
```
完整代码如下:
阅读全文
相关推荐
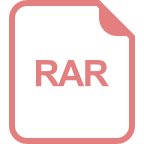
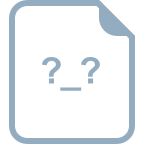
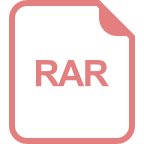
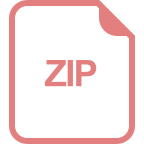
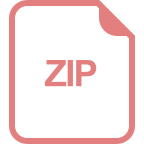
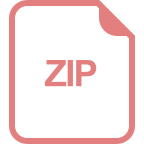
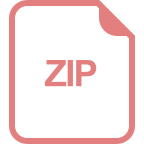
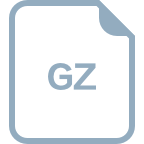
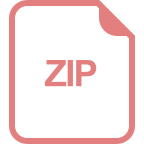
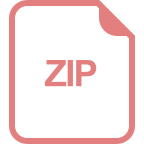
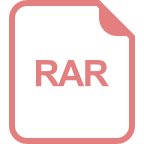
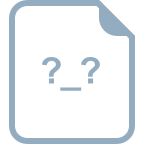
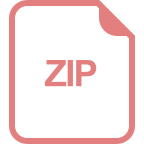