qt实现智能购票系统信号槽机制的以goupiao为类名头文件和cpp文件还有ui文件
时间: 2023-06-12 22:03:35 浏览: 206
这里给出一个简单的智能购票系统的示例代码,包含了信号槽机制和使用Qt Designer生成的UI界面。注意,这只是一个简单的示例,具体实现可能会因为需求不同而有所差异。
首先,我们需要定义一个名为 `GoUPiao` 的类,用于实现购票系统的功能。在 `GoUPiao.h` 文件中,我们可以定义如下的类:
```cpp
#ifndef GOUPIAO_H
#define GOUPIAO_H
#include <QWidget>
namespace Ui {
class GoUPiao;
}
class GoUPiao : public QWidget
{
Q_OBJECT
public:
explicit GoUPiao(QWidget *parent = nullptr);
~GoUPiao();
private slots:
void on_startBtn_clicked();
signals:
void startSignal();
private:
Ui::GoUPiao *ui;
};
#endif // GOUPIAO_H
```
在 `GoUPiao.cpp` 文件中,我们可以实现类的构造函数和析构函数:
```cpp
#include "GoUPiao.h"
#include "ui_GoUPiao.h"
GoUPiao::GoUPiao(QWidget *parent) :
QWidget(parent),
ui(new Ui::GoUPiao)
{
ui->setupUi(this);
}
GoUPiao::~GoUPiao()
{
delete ui;
}
```
接下来,我们需要在 `GoUPiao.ui` 文件中设计购票系统的UI界面。这个部分可以使用Qt Designer完成。在设计完成后,我们需要将UI界面转换为代码,并将代码添加到 `GoUPiao.cpp` 文件中:
```cpp
#include "GoUPiao.h"
#include "ui_GoUPiao.h"
GoUPiao::GoUPiao(QWidget *parent) :
QWidget(parent),
ui(new Ui::GoUPiao)
{
ui->setupUi(this);
// 绑定信号与槽
connect(ui->startBtn, SIGNAL(clicked()), this, SIGNAL(startSignal()));
}
GoUPiao::~GoUPiao()
{
delete ui;
}
void GoUPiao::on_startBtn_clicked()
{
// 处理开始购票的逻辑
}
```
在上面的代码中,我们使用 `connect()` 函数将 `startBtn` 的 `clicked()` 信号与 `GoUPiao` 类的 `startSignal()` 信号绑定起来。这样,当用户点击“开始”按钮时,就会发出 `startSignal` 信号,从而触发购票系统的后续操作。
最后,我们需要在主程序中创建 `GoUPiao` 类的实例,并将其添加到主窗口中。这部分代码可以类似于下面这样:
```cpp
#include <QApplication>
#include <QMainWindow>
#include "GoUPiao.h"
int main(int argc, char *argv[])
{
QApplication a(argc, argv);
// 创建主窗口
QMainWindow mainWindow;
// 创建购票系统
GoUPiao goUPiao(&mainWindow);
// 将购票系统添加到主窗口中
mainWindow.setCentralWidget(&goUPiao);
// 显示主窗口
mainWindow.show();
return a.exec();
}
```
在上面的代码中,我们首先创建了主窗口,然后创建了 `GoUPiao` 类的实例,并将其添加到主窗口中。最后,我们调用 `mainWindow.show()` 函数来显示主窗口,并调用 `a.exec()` 函数来启动Qt应用程序的事件循环。
这样,我们就完成了一个简单的智能购票系统的实现,其中包含了信号槽机制和使用Qt Designer生成的UI界面。
阅读全文
相关推荐
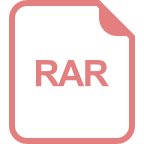
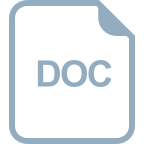
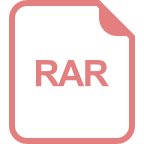
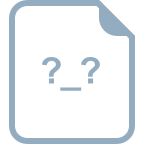
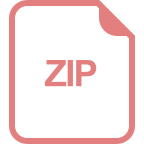
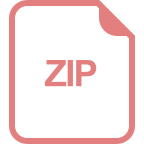