vue 如何做动态路由菜单
时间: 2023-07-20 08:32:17 浏览: 122
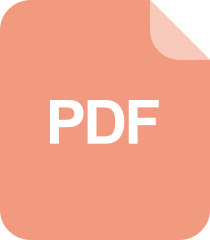
Vue 动态添加路由及生成菜单的方法示例
要实现动态路由菜单,需要以下几个步骤:
1. 定义路由配置
首先,需要定义路由配置。可以将路由配置存储在一个数组或者数据库中,并动态生成路由。例如:
```
const routes = [
{
path: '/home',
name: 'Home',
component: Home
},
{
path: '/about',
name: 'About',
component: About
},
// ...
]
```
2. 获取路由配置
然后,需要获取路由配置。这可以通过异步请求后端接口获取路由配置数据,或者将路由配置存储在 Vuex 中。例如:
```
const store = new Vuex.Store({
state: {
routes: [] // 存储路由配置
},
mutations: {
setRoutes(state, routes) {
state.routes = routes
}
},
actions: {
async fetchRoutes({ commit }) {
// 异步请求获取路由配置
const response = await axios.get('/api/routes')
commit('setRoutes', response.data)
}
}
})
```
3. 动态生成路由
接下来,需要动态生成路由。这可以通过在路由配置数组中循环,调用 `router.addRoute` 方法来实现。例如:
```
const router = createRouter({
history: createWebHistory(),
routes: []
})
store.watch(
state => state.routes,
(routes) => {
router.getRoutes().forEach(route => {
// 删除旧路由
router.removeRoute(route.name)
})
routes.forEach(route => {
// 添加新路由
router.addRoute(route.name, {
path: route.path,
name: route.name,
component: () => import(`@/views/${route.component}.vue`)
})
})
},
{ immediate: true }
)
```
4. 渲染菜单
最后,需要根据路由配置来渲染菜单。可以使用 `v-for` 循环渲染动态路由菜单。例如:
```
<template>
<div>
<ul>
<li v-for="route in routes" :key="route.name">
<router-link :to="route.path">{{ route.name }}</router-link>
</li>
</ul>
<router-view />
</div>
</template>
<script>
import { mapState } from 'vuex'
export default {
computed: {
...mapState(['routes'])
}
}
</script>
```
通过以上步骤,就可以实现动态路由菜单了。
阅读全文
相关推荐
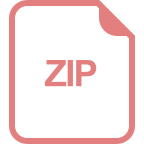








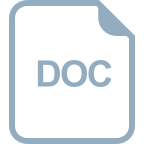






