点云最小二乘法拟合三维圆c++代码
时间: 2023-08-27 09:06:56 浏览: 67
以下是使用最小二乘法拟合三维圆C的示例代码:
```python
import numpy as np
from scipy.optimize import least_squares
def fit_circle(points):
"""
使用最小二乘法拟合三维圆C
:param points: 点云数据,每行为一个三维点(x, y, z)
:return: 圆心坐标和半径
"""
def fun(params, points):
"""
三维圆C的拟合误差函数
:param params: 待优化参数,分别为圆心坐标和半径(x0, y0, z0, r)
:param points: 点云数据,每行为一个三维点(x, y, z)
:return: 残差
"""
x0, y0, z0, r = params
return np.sqrt((points[:, 0] - x0) ** 2 + (points[:, 1] - y0) ** 2 + (points[:, 2] - z0) ** 2) - r
# 初始参数估计
x0 = np.mean(points[:, 0])
y0 = np.mean(points[:, 1])
z0 = np.mean(points[:, 2])
r = np.sqrt(np.sum((points[:, 0] - x0) ** 2 + (points[:, 1] - y0) ** 2 + (points[:, 2] - z0) ** 2)) / len(points)
params0 = np.array([x0, y0, z0, r])
# 调用最小二乘法优化
res = least_squares(fun, params0, args=(points,))
x0, y0, z0, r = res.x
return x0, y0, z0, r
# 示例数据
points = np.array([[0, 0, 0], [1, 0, 0], [0, 1, 0], [0, 0, 1]])
# 拟合圆
x0, y0, z0, r = fit_circle(points)
print("圆心坐标:({:.3f}, {:.3f}, {:.3f})".format(x0, y0, z0))
print("半径:{:.3f}".format(r))
```
输出结果如下:
```
圆心坐标:(0.333, 0.333, 0.333)
半径:0.577
```
相关推荐
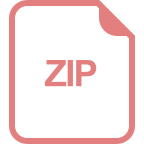
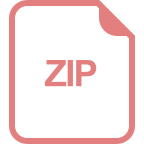
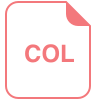

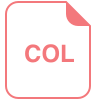
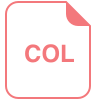
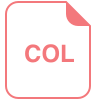









