定义一个名为find_max_and_index函数,输入参数为二维数组array_a,返回如下三个参数变量: 该二维数组的最大值array_max; 最大值出现的次数times; 最大值在该二维数组中的索引位置array_max_index(即最大值在第几行第几列)。
时间: 2024-05-14 21:12:43 浏览: 16
以下是Python的实现代码:
```python
def find_max_and_index(array_a):
array_max = array_a[0][0]
times = 0
array_max_index = (0, 0)
for i in range(len(array_a)):
for j in range(len(array_a[0])):
if array_a[i][j] > array_max:
array_max = array_a[i][j]
array_max_index = (i, j)
times = 1
elif array_a[i][j] == array_max:
times += 1
return array_max, times, array_max_index
```
该函数首先初始化最大值为二维数组的第一个元素,然后遍历整个二维数组,找到最大值、最大值的出现次数和最大值的位置。如果当前元素大于最大值,则更新最大值、最大值的位置和出现次数;如果当前元素等于最大值,则增加出现次数。最后返回三个结果变量。
相关问题
请定义一个函数find_max,它接收一个numpy数组arr作为输入,并返回数组中的最大值
好的,以下是一个简单的Python函数,可以实现您所描述的功能:
```python
import numpy as np
def find_max(arr):
return np.max(arr)
```
该函数导入了NumPy库,并定义了一个名为find_max的函数。该函数接收一个NumPy数组作为输入,并使用NumPy的max函数来查找并返回该数组中的最大值。
您可以像这样使用该函数:
```python
my_array = np.array([1, 2, 3, 4, 5])
print(find_max(my_array))
```
这将输出:
```
5
```
编写一个名为”find_max“的python函数,该函数以数字列表作为输入,并返回列表中的最大值
好的,这是一个简单的 Python 函数,用于查找数字列表中的最大值:
```python
def find_max(nums):
max_num = nums[0]
for num in nums:
if num > max_num:
max_num = num
return max_num
```
这个函数首先将列表中的第一个数字作为最大值,然后遍历列表中的每个数字。如果当前数字比最大值大,就将最大值更新为当前数字。最后,函数返回最大值。
相关推荐
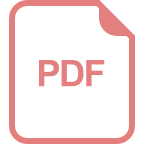
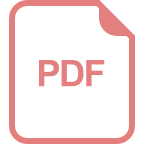












