有详细的代码说明么
时间: 2023-08-06 15:04:59 浏览: 92
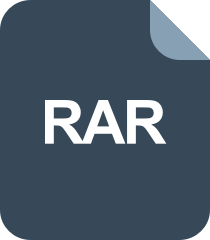
详细说明和代码
以下是一个简单的代码示例,演示了如何使用 ThinkAdmin 前端与后端进行数据交互:
前端代码(使用 jQuery 发送 Ajax 请求):
```javascript
// 发送 GET 请求获取数据
$.ajax({
url: '/api/get_data',
type: 'GET',
data: {
// 请求参数
id: 1
},
dataType: 'json',
success: function(resp) {
// 处理返回的数据
console.log(resp);
},
error: function(xhr, status, error) {
// 处理请求错误
console.error(error);
}
});
// 发送 POST 请求保存数据
$.ajax({
url: '/api/save_data',
type: 'POST',
data: {
// 请求参数
id: 1,
name: '张三'
},
dataType: 'json',
success: function(resp) {
// 处理返回的数据
console.log(resp);
},
error: function(xhr, status, error) {
// 处理请求错误
console.error(error);
}
});
```
后端代码(使用 ThinkPHP 提供的 API 接口):
```php
<?php
namespace app\api\controller;
use think\Controller;
class MyApi extends Controller {
/**
* 获取数据接口
*/
public function getData() {
$id = $this->request->param('id');
// 根据 ID 获取数据
$data = [
'id' => $id,
'name' => '张三'
];
// 返回 JSON 格式的数据
return json($data);
}
/**
* 保存数据接口
*/
public function saveData() {
$id = $this->request->param('id');
$name = $this->request->param('name');
// 根据 ID 保存数据
$result = true;
// 返回 JSON 格式的数据
return json(['success' => $result]);
}
}
```
在上面的示例中,前端使用 jQuery 发送了 GET 和 POST 请求,分别请求了获取数据接口和保存数据接口。后端使用 ThinkPHP 提供的 API 接口,分别处理了获取数据和保存数据的请求,并返回 JSON 格式的数据给前端。
需要注意的是,在实际开发中,需要根据具体的业务需求和系统架构设计 API 接口,同时需要进行安全性和性能优化等方面的考虑。
阅读全文
相关推荐
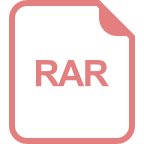
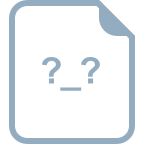
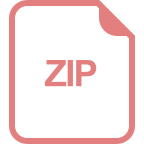
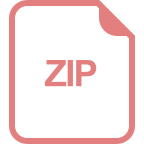
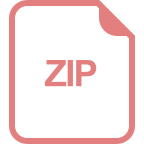
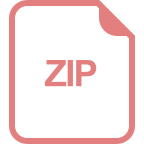
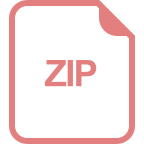
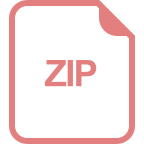
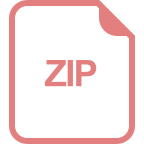
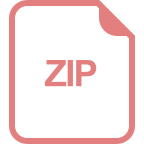
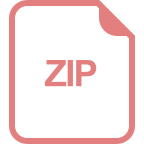
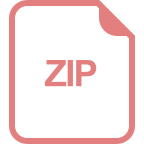