返回series bool值中为true的索引
时间: 2024-03-03 12:51:18 浏览: 261
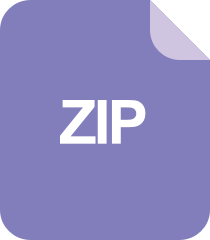
yy.zip_bool insert
你可以使用 `pandas.Series` 的 `index` 属性和布尔索引来实现。例如,假设你的 `Series` 名称为 `s`,你可以使用以下代码返回 `s` 中为 `True` 的索引:
```python
s[s == True].index
```
其中,`s == True` 返回一个布尔类型的 `Series`,其中为 `True` 的位置表示 `s` 中对应位置为 `True`。然后,我们使用布尔索引的方式提取 `s` 中为 `True` 的值,最后再通过 `index` 属性返回它们的索引。
阅读全文
相关推荐
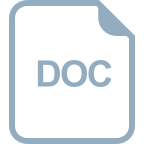
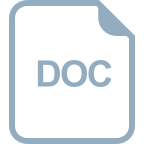

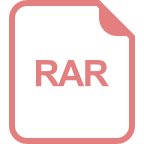
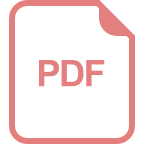
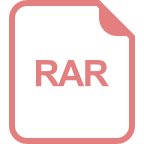
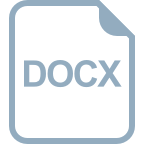
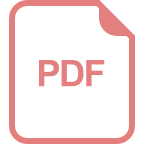
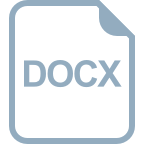
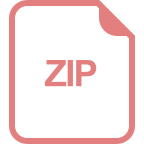
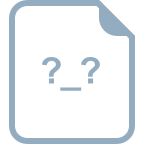
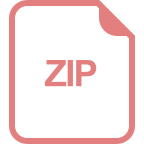
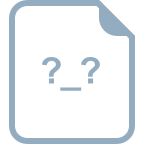
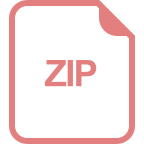
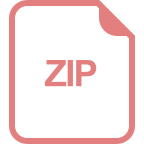
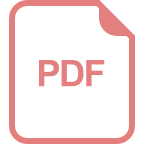
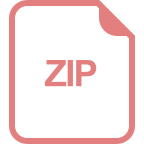