嵌入式Linux如何用C读写spi
时间: 2024-06-10 22:10:49 浏览: 296
在嵌入式Linux中,可以使用C语言的SPI库来读写SPI。下面是一个简单的示例程序:
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <fcntl.h>
#include <string.h>
#include <linux/spi/spidev.h>
#define SPI_DEVICE "/dev/spidev0.0"
#define SPI_MODE SPI_MODE_0
#define SPI_BITS_PER_WORD 8
#define SPI_SPEED_HZ 1000000
int main(int argc, char* argv[])
{
int fd;
unsigned char tx[3];
unsigned char rx[3];
fd = open(SPI_DEVICE, O_RDWR);
if (fd < 0) {
perror("Failed to open SPI device");
return 1;
}
// Configure SPI mode, bits per word, and clock speed
if (ioctl(fd, SPI_IOC_WR_MODE, &SPI_MODE) < 0) {
perror("Failed to set SPI mode");
return 1;
}
if (ioctl(fd, SPI_IOC_WR_BITS_PER_WORD, &SPI_BITS_PER_WORD) < 0) {
perror("Failed to set SPI bits per word");
return 1;
}
if (ioctl(fd, SPI_IOC_WR_MAX_SPEED_HZ, &SPI_SPEED_HZ) < 0) {
perror("Failed to set SPI speed");
return 1;
}
// Write data to SPI device
memset(tx, 0, sizeof(tx));
memset(rx, 0, sizeof(rx));
tx[0] = 0x01;
tx[1] = 0x02;
tx[2] = 0x03;
if (write(fd, tx, sizeof(tx)) != sizeof(tx)) {
perror("Failed to write to SPI device");
return 1;
}
// Read data from SPI device
if (read(fd, rx, sizeof(rx)) != sizeof(rx)) {
perror("Failed to read from SPI device");
return 1;
}
// Print received data
printf("Received: 0x%02x 0x%02x 0x%02x\n", rx[0], rx[1], rx[2]);
close(fd);
return 0;
}
```
以上代码示例使用了Linux内核提供的`spidev`设备驱动程序,通过`ioctl()`函数来配置SPI模式、字长和时钟速率。然后,通过`write()`函数向SPI设备写入数据,通过`read()`函数从SPI设备读取数据,最后将读取的数据打印出来。
注意:在运行该程序之前,需要先确认系统中是否已经加载了`spidev`驱动程序,如果没有,需要先加载该驱动程序。另外,需要根据实际硬件连接情况修改代码中的SPI设备节点、SPI模式、字长和时钟速率等参数。
阅读全文
相关推荐














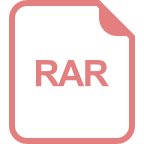

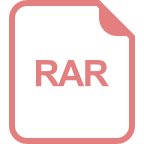