基于stm32室内环境检测系统代码
时间: 2023-11-15 09:06:04 浏览: 37
由于室内环境检测系统需要检测的参数较多,例如温度、湿度、气压、CO2浓度等,所以需要使用多种传感器进行数据采集。以下是一份基于STM32的室内环境检测系统的代码,仅供参考:
```c
#include "stdio.h"
#include "stdlib.h"
#include "string.h"
#include "stm32f10x.h"
#include "delay.h"
#include "usart.h"
#include "i2c.h"
#define TEMP_ADDR 0xD0 // 温度传感器I2C地址
#define HUM_ADDR 0x80 // 湿度传感器I2C地址
#define PRESS_ADDR 0xEE // 气压传感器I2C地址
#define CO2_ADDR 0x68 // CO2传感器I2C地址
float temp, hum, press, co2;
/**
* @brief I2C读取数据
* @param I2Cx: I2C外设
* @param DevAddr: 设备地址
* @param RegAddr: 寄存器地址
* @param pBuffer: 数据缓冲区
* @param NumByteToRead: 数据长度
* @retval None
*/
void I2C_ReadData(I2C_TypeDef* I2Cx, uint8_t DevAddr, uint8_t RegAddr, uint8_t* pBuffer, uint16_t NumByteToRead)
{
/*!< While the bus is busy */
while (I2C_GetFlagStatus(I2Cx, I2C_FLAG_BUSY));
/*!< Send START condition */
I2C_GenerateSTART(I2Cx, ENABLE);
/*!< Test on EV5 and clear it */
while (!I2C_CheckEvent(I2Cx, I2C_EVENT_MASTER_MODE_SELECT));
/*!< Send device address for write */
I2C_Send7bitAddress(I2Cx, DevAddr, I2C_Direction_Transmitter);
/*!< Test on EV6 and clear it */
while (!I2C_CheckEvent(I2Cx, I2C_EVENT_MASTER_TRANSMITTER_MODE_SELECTED));
/*!< Send the device's internal address to write to */
I2C_SendData(I2Cx, RegAddr);
/*!< Test on EV8 and clear it */
while (!I2C_CheckEvent(I2Cx, I2C_EVENT_MASTER_BYTE_TRANSMITTED));
/*!< Send START condition a second time */
I2C_GenerateSTART(I2Cx, ENABLE);
/*!< Test on EV5 and clear it */
while (!I2C_CheckEvent(I2Cx, I2C_EVENT_MASTER_MODE_SELECT));
/*!< Send device address for read */
I2C_Send7bitAddress(I2Cx, DevAddr, I2C_Direction_Receiver);
/*!< Test on EV6 and clear it */
while (!I2C_CheckEvent(I2Cx, I2C_EVENT_MASTER_RECEIVER_MODE_SELECTED));
/*!< While there is data to be read */
while (NumByteToRead)
{
if (NumByteToRead == 1)
{
/*!< Disable Acknowledgement */
I2C_AcknowledgeConfig(I2Cx, DISABLE);
/*!< Send STOP Condition */
I2C_GenerateSTOP(I2Cx, ENABLE);
}
/*!< Test on EV7 and clear it */
if (I2C_CheckEvent(I2Cx, I2C_EVENT_MASTER_BYTE_RECEIVED))
{
/*!< Read a byte from the device */
*pBuffer = I2C_ReceiveData(I2Cx);
/*!< Point to the next location where the byte read will be saved */
pBuffer++;
/*!< Decrement the read bytes counter */
NumByteToRead--;
}
}
/*!< Enable Acknowledgement to be ready for another reception */
I2C_AcknowledgeConfig(I2Cx, ENABLE);
}
/**
* @brief I2C写入数据
* @param I2Cx: I2C外设
* @param DevAddr: 设备地址
* @param RegAddr: 寄存器地址
* @param pBuffer: 数据缓冲区
* @param NumByteToWrite: 数据长度
* @retval None
*/
void I2C_WriteData(I2C_TypeDef* I2Cx, uint8_t DevAddr, uint8_t RegAddr, uint8_t* pBuffer, uint16_t NumByteToWrite)
{
/*!< While the bus is busy */
while (I2C_GetFlagStatus(I2Cx, I2C_FLAG_BUSY));
/*!< Send START condition */
I2C_GenerateSTART(I2Cx, ENABLE);
/*!< Test on EV5 and clear it */
while (!I2C_CheckEvent(I2Cx, I2C_EVENT_MASTER_MODE_SELECT));
/*!< Send device address for write */
I2C_Send7bitAddress(I2Cx, DevAddr, I2C_Direction_Transmitter);
/*!< Test on EV6 and clear it */
while (!I2C_CheckEvent(I2Cx, I2C_EVENT_MASTER_TRANSMITTER_MODE_SELECTED));
/*!< Send the device's internal address to write to */
I2C_SendData(I2Cx, RegAddr);
/*!< Test on EV8 and clear it */
while (!I2C_CheckEvent(I2Cx, I2C_EVENT_MASTER_BYTE_TRANSMITTED));
/*!< Send the byte to be written */
while (NumByteToWrite--)
{
I2C_SendData(I2Cx, *pBuffer++);
/*!< Test on EV8 and clear it */
while (!I2C_CheckEvent(I2Cx, I2C_EVENT_MASTER_BYTE_TRANSMITTED));
}
/*!< Send STOP condition */
I2C_GenerateSTOP(I2Cx, ENABLE);
}
/**
* @brief 温度传感器初始化
* @param None
* @retval None
*/
void Temp_Init(void)
{
uint8_t tmp = 0x00;
I2C_WriteData(I2C1, TEMP_ADDR, 0x01, &tmp, 1);
}
/**
* @brief 读取温度传感器数据
* @param None
* @retval None
*/
void Temp_Read(void)
{
uint8_t buf[2];
I2C_ReadData(I2C1, TEMP_ADDR, 0x00, buf, 2);
temp = (float)(((buf[0] << 8) | buf[1]) >> 2) * 0.25;
}
/**
* @brief 湿度传感器初始化
* @param None
* @retval None
*/
void Hum_Init(void)
{
uint8_t tmp[2] = {0x02, 0x01};
I2C_WriteData(I2C1, HUM_ADDR, 0x00, tmp, 2);
}
/**
* @brief 读取湿度传感器数据
* @param None
* @retval None
*/
void Hum_Read(void)
{
uint8_t buf[2];
I2C_ReadData(I2C1, HUM_ADDR, 0x00, buf, 2);
hum = (float)(((buf[0] << 8) | buf[1]) * 0.00190735 - 6);
}
/**
* @brief 气压传感器初始化
* @param None
* @retval None
*/
void Press_Init(void)
{
uint8_t tmp[2] = {0x27, 0x00};
I2C_WriteData(I2C1, PRESS_ADDR, 0x26, tmp, 2);
}
/**
* @brief 读取气压传感器数据
* @param None
* @retval None
*/
void Press_Read(void)
{
uint8_t buf[3];
I2C_ReadData(I2C1, PRESS_ADDR, 0x00, buf, 3);
press = (float)(((buf[0] << 16) | (buf[1] << 8) | buf[2]) / 4096.0);
}
/**
* @brief CO2传感器初始化
* @param None
* @retval None
*/
void CO2_Init(void)
{
uint8_t tmp = 0x01;
I2C_WriteData(I2C1, CO2_ADDR, 0x01, &tmp, 1);
}
/**
* @brief 读取CO2传感器数据
* @param None
* @retval None
*/
void CO2_Read(void)
{
uint8_t buf[2];
I2C_ReadData(I2C1, CO2_ADDR, 0x08, buf, 2);
co2 = (float)(((buf[0] << 8) | buf[1]) * 0.1);
}
int main(void)
{
USART_Config();
I2C_Config();
Temp_Init();
Hum_Init();
Press_Init();
CO2_Init();
while (1)
{
Temp_Read();
Hum_Read();
Press_Read();
CO2_Read();
printf("Temp: %.2fC\tHum: %.2f%%RH\tPress: %.2fkPa\tCO2: %.2fppm\r\n", temp, hum, press, co2);
Delay_ms(1000);
}
}
```
在上述代码中,我们使用了STM32内置的I2C外设以及一些常用的函数,如I2C_ReadData()和I2C_WriteData()用于读写I2C数据。在主函数中,我们首先进行串口和I2C的配置,并对每个传感器进行初始化。然后在一个死循环中不断读取传感器数据,并通过串口输出。
需要注意的是,不同的传感器有不同的I2C地址和数据格式,因此需要根据具体的传感器手册进行配置和数据解析。此外,还需要在代码中添加对应的库文件和头文件,这里就不做展开了。
相关推荐
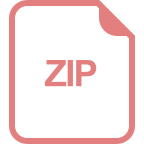
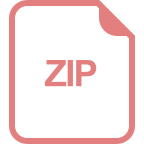














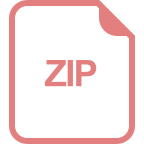