51单片机nrf24l01程序
时间: 2023-09-03 18:15:03 浏览: 58
以下是51单片机使用nrf24l01无线模块的基本程序示例:
```c
#include <reg52.h>
#include <intrins.h>
#define uchar unsigned char
#define uint unsigned int
sbit CE = P3^5; //CE引脚
sbit CSN = P3^4; //CSN引脚
void Delay_us(uint us) //微秒级延时函数
{
while(us--)
{
_nop_();
_nop_();
_nop_();
_nop_();
}
}
void Delay_ms(uint ms) //毫秒级延时函数
{
while(ms--)
{
Delay_us(1000);
}
}
void SPI_ByteSend(uchar dat) //SPI发送一个字节函数
{
uchar i;
for(i=0; i<8; i++)
{
SCK = 0;
MOSI = dat & 0x80;
dat <<= 1;
SCK = 1;
}
}
uchar SPI_ByteRead(void) //SPI读取一个字节函数
{
uchar i, dat;
for(i=0; i<8; i++)
{
dat <<= 1;
SCK = 0;
if(MISO)
{
dat |= 0x01;
}
SCK = 1;
}
return dat;
}
void NRF24L01_WriteReg(uchar addr, uchar dat) //写寄存器函数
{
CE = 0;
CSN = 0;
SPI_ByteSend(addr | 0x20);
SPI_ByteSend(dat);
CSN = 1;
}
uchar NRF24L01_ReadReg(uchar addr) //读寄存器函数
{
uchar dat;
CE = 0;
CSN = 0;
SPI_ByteSend(addr);
dat = SPI_ByteRead();
CSN = 1;
return dat;
}
void NRF24L01_WriteBuf(uchar addr, uchar *pBuf, uchar len) //写缓冲区函数
{
uchar i;
CE = 0;
CSN = 0;
SPI_ByteSend(addr | 0x20);
for(i=0; i<len; i++)
{
SPI_ByteSend(*(pBuf+i));
}
CSN = 1;
}
void NRF24L01_ReadBuf(uchar addr, uchar *pBuf, uchar len) //读缓冲区函数
{
uchar i;
CE = 0;
CSN = 0;
SPI_ByteSend(addr);
for(i=0; i<len; i++)
{
*(pBuf+i) = SPI_ByteRead();
}
CSN = 1;
}
void NRF24L01_TxPacket(uchar *pBuf, uchar len) //发射数据包函数
{
CE = 0;
CSN = 0;
SPI_ByteSend(0xA0);
for(i=0; i<len; i++)
{
SPI_ByteSend(*(pBuf+i));
}
CSN = 1;
CE = 1;
Delay_us(10);
CE = 0;
}
void NRF24L01_RxPacket(uchar *pBuf) //接收数据包函数
{
CE = 1;
Delay_us(10);
CE = 0;
CSN = 0;
SPI_ByteSend(0x61);
for(i=0; i<32; i++)
{
*(pBuf+i) = SPI_ByteRead();
}
CSN = 1;
}
void NRF24L01_Init(void) //初始化函数
{
uchar i;
NRF24L01_WriteReg(0x00, 0x0A); //配置寄存器,开启CRC校验,16位
NRF24L01_WriteReg(0x01, 0x03); //配置寄存器,发射模式,最高发射功率
NRF24L01_WriteReg(0x02, 0x03); //配置寄存器,2Mbps数据传输速率,5个字节的地址宽度
NRF24L01_WriteReg(0x03, 0x00); //配置寄存器,关闭自动重发,自动ACK功能
NRF24L01_WriteReg(0x04, 0x0F); //配置寄存器,接收通道0的地址为0x0F0F0F0F0E
NRF24L01_WriteReg(0x05, 0x0E);
NRF24L01_WriteReg(0x06, 0x0F);
NRF24L01_WriteReg(0x07, 0x0F);
NRF24L01_WriteReg(0x11, 0x20); //配置寄存器,16MHz晶振,低噪音增益模式
CE = 0;
CSN = 1;
Delay_ms(10);
NRF24L01_WriteReg(0x00, 0x0F); //配置寄存器,开启接收模式
CE = 1;
}
void main()
{
uchar i, buf[32];
NRF24L01_Init();
while(1)
{
if(NRF24L01_ReadReg(0x07) & 0x40) //检测数据包是否接收完成
{
NRF24L01_RxPacket(buf);
for(i=0; i<32; i++)
{
putchar(buf[i]); //输出接收到的数据
}
printf("\r\n");
NRF24L01_WriteReg(0x07, 0x40); //清除中断标志位
}
}
}
```
注意:在程序中需要根据具体的硬件电路来定义CE、CSN、MOSI、MISO、SCK等引脚,以及使用的延时函数。
相关推荐
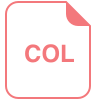
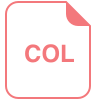
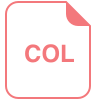
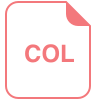
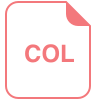









