分页怎么通过mybaits和vue实现
时间: 2024-05-10 11:18:31 浏览: 13
在使用 MyBatis 和 Vue 实现分页功能,一般需要以下步骤:
1. 在后端使用 MyBatis 实现分页查询。可以使用 MyBatis 提供的分页插件 PageHelper,也可以手动编写 SQL 语句实现分页查询。
2. 在前端使用 Vue 实现分页组件。可以使用第三方的分页组件库,比如 Element UI、Bootstrap Vue 等,也可以自己编写分页组件。
3. 前端向后端发送分页请求。可以通过 Ajax 发送 GET 请求,请求参数中包含当前页码、每页显示数量等信息。
4. 后端接收请求,进行分页查询并返回结果。返回的结果中包含当前页数据、总数据量、总页数等信息。
5. 前端接收到结果,更新分页组件显示的页码和数据。
下面是一个简单的示例代码,仅供参考:
MyBatis 分页查询代码:
```java
public interface UserMapper {
// 分页查询用户信息
List<User> getUserListByPage(@Param("offset") int offset, @Param("pageSize") int pageSize);
}
// 使用 PageHelper 分页插件的示例代码
PageHelper.startPage(pageNum, pageSize);
List<User> userList = userMapper.getUserListByPage();
PageInfo<User> pageInfo = new PageInfo<>(userList);
// 手动编写 SQL 分页查询的示例代码
List<User> userList = userMapper.getUserListByPage(offset, pageSize);
int totalCount = userMapper.getUserCount();
int totalPage = (totalCount + pageSize - 1) / pageSize;
```
Vue 分页组件代码:
```html
<template>
<div>
<table>
<thead>
<tr>
<th>姓名</th>
<th>年龄</th>
<th>性别</th>
</tr>
</thead>
<tbody>
<tr v-for="user in userList" :key="user.id">
<td>{{ user.name }}</td>
<td>{{ user.age }}</td>
<td>{{ user.gender }}</td>
</tr>
</tbody>
</table>
<div class="pagination">
<ul>
<li v-if="currentPage > 1" @click="changePage(currentPage - 1)">«</li>
<li v-for="page in pages" :key="page"
:class="{ active: page === currentPage }"
@click="changePage(page)">
{{ page }}
</li>
<li v-if="currentPage < totalPages" @click="changePage(currentPage + 1)">»</li>
</ul>
</div>
</div>
</template>
<script>
export default {
data() {
return {
userList: [],
currentPage: 1,
pageSize: 10,
totalCount: 0,
};
},
computed: {
totalPages() {
return Math.ceil(this.totalCount / this.pageSize);
},
pages() {
let startPage = Math.max(1, this.currentPage - 2);
let endPage = Math.min(this.totalPages, this.currentPage + 2);
let pages = [];
for (let i = startPage; i <= endPage; i++) {
pages.push(i);
}
return pages;
},
},
methods: {
changePage(page) {
this.currentPage = page;
this.getUserList();
},
getUserList() {
axios.get('/api/user', {
params: {
pageNum: this.currentPage,
pageSize: this.pageSize,
},
}).then(res => {
this.userList = res.data.list;
this.totalCount = res.data.totalCount;
});
},
},
mounted() {
this.getUserList();
},
};
</script>
```
相关推荐
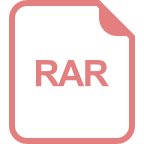
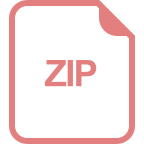
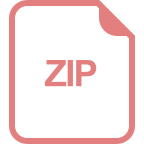














