利用pyqt5显示yolov5的检测结果
时间: 2023-07-10 09:11:13 浏览: 80
好的,可以按照以下步骤进行:
1. 首先,导入PyQt5和YoloV5的相关库和模块,以及必要的函数和变量。例如:
```python
import sys
import cv2
import numpy as np
from PyQt5.QtGui import QImage, QPixmap
from PyQt5.QtWidgets import QApplication, QLabel, QWidget, QHBoxLayout
from PyQt5.QtCore import Qt, QTimer
# 导入yolov5模型和配置
sys.path.append('yolov5')
from models.experimental import attempt_load
from utils.general import check_img_size, non_max_suppression, scale_coords, xyxy2xywh
from utils.datasets import letterbox
from utils.plots import plot_one_box, plot_results
from utils.torch_utils import select_device
```
2. 然后,创建一个自定义QWidget,用于显示图像和检测结果。例如:
```python
class YoloWidget(QWidget):
def __init__(self):
super().__init__()
self.image_label = QLabel(self)
self.result_label = QLabel(self)
hbox = QHBoxLayout()
hbox.addWidget(self.image_label)
hbox.addWidget(self.result_label)
self.setLayout(hbox)
self.setWindowTitle('YoloV5 Demo')
self.setGeometry(100, 100, 1200, 600)
# 加载yolov5模型
self.device = select_device('')
self.model = attempt_load('yolov5s.pt', map_location=self.device)
self.names = self.model.module.names if hasattr(self.model, 'module') else self.model.names
self.colors = [[np.random.randint(0, 255) for _ in range(3)] for _ in range(len(self.names))]
```
在这个例子中,我们创建了一个名为`YoloWidget`的自定义QWidget,它包含两个QLabel控件,一个用于显示图像,另一个用于显示检测结果。我们使用QHBoxLayout将它们水平排列,并将其设置为QWidget的布局。我们还加载YoloV5模型,并使用`select_device`函数选择设备。
3. 在`YoloWidget`类中,编写一个名为`detect_objects`的函数,用于对指定图像进行检测并显示结果。例如:
```python
def detect_objects(self, image_path):
# 加载图像并调整大小
img0 = cv2.imread(image_path)
img = letterbox(img0, new_shape=640)[0]
img = img[:, :, ::-1].transpose(2, 0, 1)
img = np.ascontiguousarray(img)
# 模型推理
img = torch.from_numpy(img).to(self.device)
img /= 255.0
if img.ndimension() == 3:
img = img.unsqueeze(0)
pred = self.model(img)[0]
pred = non_max_suppression(pred, 0.4, 0.5)
# 绘制检测结果
results = []
for i, det in enumerate(pred):
if len(det):
det[:, :4] = scale_coords(img.shape[2:], det[:, :4], img0.shape).round()
for *xyxy, conf, cls in reversed(det):
c = int(cls)
label = f'{self.names[c]} {conf:.2f}'
plot_one_box(xyxy, img0, label=label, color=self.colors[c], line_thickness=3)
# 将检测结果保存到列表中
results.append({
'class': self.names[c],
'confidence': float(conf),
'x': int(xyxy[0]),
'y': int(xyxy[1]),
'w': int(xyxy[2] - xyxy[0]),
'h': int(xyxy[3] - xyxy[1])
})
# 显示图像和检测结果
height, width, channel = img0.shape
bytesPerLine = 3 * width
qimage = QImage(img0.data, width, height, bytesPerLine, QImage.Format_RGB888)
pixmap = QPixmap.fromImage(qimage)
self.image_label.setPixmap(pixmap)
self.image_label.resize(pixmap.width(), pixmap.height())
result_img = plot_results(pred, img0, self.names, self.colors, line_thickness=3)
result_qimage = QImage(result_img.data, result_img.shape[1], result_img.shape[0], QImage.Format_RGB888)
result_pixmap = QPixmap.fromImage(result_qimage)
self.result_label.setPixmap(result_pixmap)
self.result_label.resize(result_pixmap.width(), result_pixmap.height())
return results
```
在这个例子中,我们使用YoloV5模型对图像进行推理,并将检测结果绘制在原始图像上。然后,我们将原始图像和检测结果分别显示在两个QLabel控件中。我们还将检测结果保存到一个列表中,并返回它。
4. 最后,在主程序中,创建一个`YoloWidget`对象并显示它。例如:
```python
if __name__ == '__main__':
app = QApplication(sys.argv)
w = YoloWidget()
w.detect_objects('image.jpg')
w.show()
sys.exit(app.exec_())
```
在这个例子中,我们创建了一个名为`w`的`YoloWidget`对象,并使用`detect_objects`函数对指定图像进行检测和显示。然后,我们将这个对象显示在屏幕上。
相关推荐
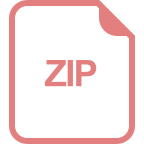
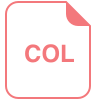
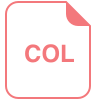
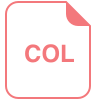
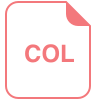
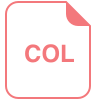









