AVR64EA48编写lcd1602的程序
时间: 2023-09-02 16:10:12 浏览: 119
下面是一个使用AVR64EA48微控制器编写LCD1602程序的示例:
```c
#include <avr/io.h>
#include <util/delay.h>
#define LCD_DATA_PORT PORTA
#define LCD_CTRL_PORT PORTB
#define LCD_RS PB0
#define LCD_RW PB1
#define LCD_EN PB2
void lcd_command(unsigned char cmd) {
LCD_DATA_PORT = cmd;
LCD_CTRL_PORT &= ~(1 << LCD_RS); // RS = 0 for command mode
LCD_CTRL_PORT &= ~(1 << LCD_RW); // RW = 0 for write mode
LCD_CTRL_PORT |= (1 << LCD_EN); // EN = 1 to enable the LCD
_delay_ms(1);
LCD_CTRL_PORT &= ~(1 << LCD_EN); // EN = 0 to disable the LCD
_delay_ms(1);
}
void lcd_data(unsigned char data) {
LCD_DATA_PORT = data;
LCD_CTRL_PORT |= (1 << LCD_RS); // RS = 1 for data mode
LCD_CTRL_PORT &= ~(1 << LCD_RW); // RW = 0 for write mode
LCD_CTRL_PORT |= (1 << LCD_EN); // EN = 1 to enable the LCD
_delay_ms(1);
LCD_CTRL_PORT &= ~(1 << LCD_EN); // EN = 0 to disable the LCD
_delay_ms(1);
}
void lcd_init() {
lcd_command(0x38); // 2 lines, 5x7 matrix
lcd_command(0x0C); // display on, cursor off
lcd_command(0x06); // increment cursor
lcd_command(0x01); // clear display
}
void lcd_clear() {
lcd_command(0x01); // clear display
}
void lcd_set_cursor(uint8_t row, uint8_t col) {
uint8_t row_offsets[] = {0x00, 0x40};
lcd_command(0x80 | (row_offsets[row] + col));
}
void lcd_write_string(const char* str) {
while (*str) {
lcd_data(*str++);
}
}
int main() {
// 初始化I/O端口
DDRA = 0xFF; // 将PORTA设置为输出模式
DDRB = 0x07; // 将PB0、PB1、PB2设置为输出模式
// 初始化LCD
lcd_init();
// 显示温湿度信息
lcd_set_cursor(0, 0);
lcd_write_string("Temperature:");
lcd_set_cursor(1, 0);
lcd_write_string("Humidity:");
while (1) {
// 获取温湿度数据,并将其转换为字符串
int temperature = read_temperature();
int humidity = read_humidity();
char temperature_str[10];
char humidity_str[10];
sprintf(temperature_str, "%d C", temperature);
sprintf(humidity_str, "%d %%", humidity);
// 更新LCD显示
lcd_set_cursor(0, 12);
lcd_write_string(temperature_str);
lcd_set_cursor(1, 10);
lcd_write_string(humidity_str);
_delay_ms(1000);
}
return 0;
}
```
请注意,上述代码仅为示例,其中的`read_temperature()`和`read_humidity()`函数需要根据具体的硬件和传感器进行实现。此外,你可能需要根据你的硬件连接情况进行相应的调整。
希望这个示例程序对你有所帮助!
阅读全文
相关推荐
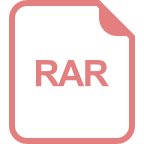
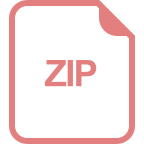
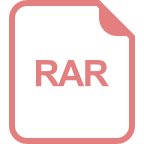
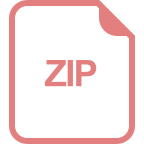
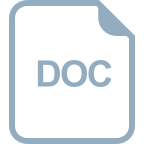
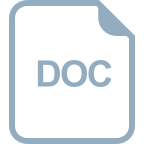
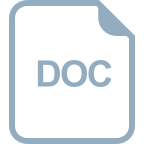
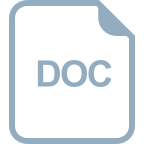
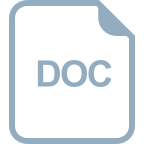
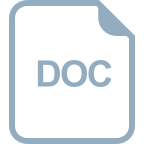
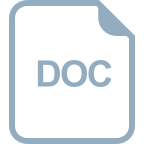
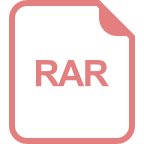
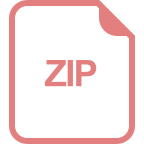