C++ cv::imwrite返回值
时间: 2023-06-28 17:12:02 浏览: 460
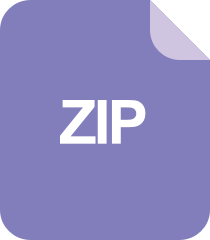
xiangsu.zip_c++像素_图像赋值
C++函数`cv::imwrite`的返回值是一个布尔值,用于表示图像是否成功保存。保存成功,则返回`true`,否则返回`false`。如果返回`false`可以使用`cv::imwrite`函数提供的错误信息来确定错误的原因。例如,可以使用以下代码来检查`cv::imwrite`函数是否成功保存了图像:
```c++
if (!cv::imwrite("output.jpg", image)) {
std::cerr << "Failed to save the image!" << std::endl;
}
```
这将在控制台上输出错误消息,如果保存失败,则输出“Failed to save the image!”。
阅读全文
相关推荐
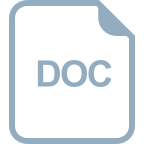
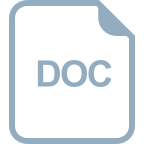
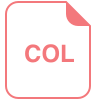
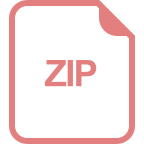
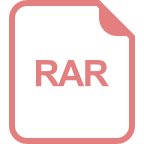
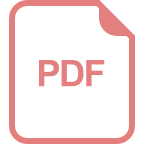
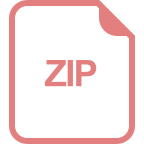
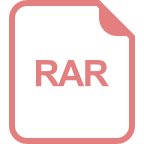
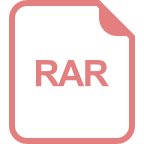
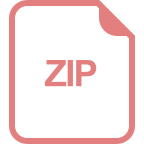
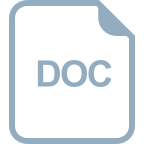
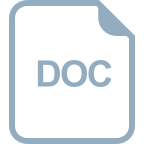
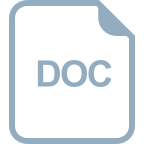
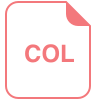
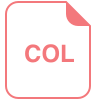



