用Java编写代码,将study文件夹中的数据恢复到study数据表中,注意删除重复数据
时间: 2023-09-08 11:05:22 浏览: 68
### 回答1:
以下是Java代码的示例,用于将study文件夹中的数据恢复到study数据表中,并删除重复数据:
```
import java.io.File;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.util.Scanner;
public class RestoreData {
public static void main(String[] args) {
Connection connection = null;
PreparedStatement statement = null;
ResultSet resultSet = null;
try {
// 加载JDBC驱动程序
Class.forName("com.mysql.jdbc.Driver");
// 创建数据库连接
connection = DriverManager.getConnection("jdbc:mysql://localhost:3306/database", "username", "password");
// 获取study文件夹中的所有文件
File folder = new File("study");
File[] files = folder.listFiles();
// 遍历所有文件,恢复数据到数据表中
for (File file : files) {
Scanner scanner = new Scanner(file);
while (scanner.hasNextLine()) {
String line = scanner.nextLine();
String[] data = line.split(",");
// 删除重复数据
statement = connection.prepareStatement("DELETE FROM study WHERE name = ?");
statement.setString(1, data[0]);
statement.executeUpdate();
// 恢复数据
statement = connection.prepareStatement("INSERT INTO study (name, score) VALUES (?,?)");
statement.setString(1, data[0]);
statement.setInt(2, Integer.parseInt(data[1]));
statement.executeUpdate();
}
scanner.close();
}
System.out.println("数据恢复成功!");
} catch (ClassNotFoundException e) {
e.printStackTrace();
} catch (SQLException e) {
e.printStackTrace();
} finally {
try {
if (resultSet != null) {
resultSet.close();
}
if (statement != null) {
statement.close();
}
if (connection != null) {
connection.close();
}
} catch (SQLException e) {
e.printStackTrace();
}
}
}
}
```
请注意,上面的代码仅供
### 回答2:
要使用Java编写代码将study文件夹中的数据恢复到study数据表中,首先需要进行一系列步骤。以下是大致的代码逻辑:
1. 创建数据库连接并打开连接,确保已经创建了study数据表。
2. 获取study文件夹中所有的文件列表。
3. 遍历文件列表,依次读取每个文件的内容。
4. 对于每个文件,首先判断study数据表中是否已存在相同的数据。
5. 如果study数据表中不存在相同的数据,则将文件内容插入到study数据表。
6. 如果study数据表中已存在相同的数据,则跳过该文件。
7. 关闭数据库连接。
以下是一个示例代码,可能需要根据实际情况进行调整:
```java
import java.io.BufferedReader;
import java.io.File;
import java.io.FileReader;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
public class StudyDataRecovery {
public static void main(String[] args) {
// 数据库连接信息
String url = "jdbc:mysql://localhost:3306/database_name";
String user = "username";
String password = "password";
// study文件夹路径
String folderPath = "study";
Connection conn = null;
PreparedStatement ps = null;
ResultSet rs = null;
try {
// 打开数据库连接
conn = DriverManager.getConnection(url, user, password);
// 获取study文件夹中所有文件列表
File folder = new File(folderPath);
File[] files = folder.listFiles();
if (files != null) {
for (File file : files) {
// 读取文件内容
StringBuilder content = new StringBuilder();
BufferedReader reader = new BufferedReader(new FileReader(file));
String line;
while ((line = reader.readLine()) != null) {
content.append(line);
}
reader.close();
// 查询study数据表是否存在相同的数据
String querySql = "SELECT * FROM study WHERE content = ?";
ps = conn.prepareStatement(querySql);
ps.setString(1, content.toString());
rs = ps.executeQuery();
// 如果不存在,则插入到study数据表中
if (!rs.next()) {
String insertSql = "INSERT INTO study (content) VALUES (?)";
ps = conn.prepareStatement(insertSql);
ps.setString(1, content.toString());
ps.executeUpdate();
System.out.println("成功插入数据:" + content.toString());
} else {
System.out.println("存在重复数据,跳过文件:" + file.getName());
}
}
}
} catch (SQLException | IOException e) {
e.printStackTrace();
} finally {
// 关闭数据库连接
try {
if (rs != null) {
rs.close();
}
if (ps != null) {
ps.close();
}
if (conn != null) {
conn.close();
}
} catch (SQLException e) {
e.printStackTrace();
}
}
}
}
```
需要注意的是,以上代码仅提供了一个大致的框架,具体实现可能根据实际情况有所不同。另外,需要将代码中的数据库连接信息、study数据表结构以及study文件夹路径等根据实际情况进行修改。
### 回答3:
使用Java编写代码,可以将study文件夹中的数据恢复到study数据表中,并确保删除重复数据。下面是一个简单的示例代码:
```java
import java.io.File;
import java.io.FileReader;
import java.io.IOException;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.PreparedStatement;
import java.sql.SQLException;
import java.util.HashSet;
import java.util.Set;
public class DataRecovery {
public static void main(String[] args) {
String folderPath = "study"; // study文件夹路径
String tableName = "study"; // study数据表名称
try {
// 连接到数据库
Connection connection = DriverManager.getConnection("jdbc:mysql://localhost:3306/database", "username", "password");
connection.setAutoCommit(false);
// 删除重复数据
PreparedStatement deleteStatement = connection.prepareStatement("DELETE FROM " + tableName + " WHERE id = ?");
Set<String> uniqueData = new HashSet<>();
// 遍历study文件夹中的文件
File folder = new File(folderPath);
if (folder.isDirectory()) {
File[] files = folder.listFiles();
for (File file : files) {
FileReader fileReader = new FileReader(file);
StringBuilder data = new StringBuilder();
int ch;
while ((ch = fileReader.read()) != -1) {
data.append((char) ch);
}
fileReader.close();
// 判断数据是否重复,如果是则删除
if (uniqueData.contains(data.toString())) {
deleteStatement.setString(1, data.toString());
deleteStatement.execute();
} else {
uniqueData.add(data.toString());
// 将数据插入数据库表中
PreparedStatement insertStatement = connection.prepareStatement("INSERT INTO " + tableName + " (data) VALUES (?)");
insertStatement.setString(1, data.toString());
insertStatement.execute();
}
}
}
// 提交事务并关闭连接
connection.commit();
connection.close();
} catch (SQLException | IOException e) {
e.printStackTrace();
}
}
}
```
以上代码示例使用了Java的JDBC(Java Database Connectivity)接口来连接数据库,并使用了MySQL数据库作为示例。请根据实际情况修改数据库连接URL、用户名和密码。在实际使用时,还需要根据具体的数据表结构修改SQL语句。代码中使用HashSet来存储唯一的数据,确保不会插入重复数据。
相关推荐
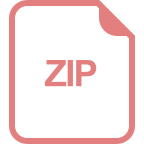
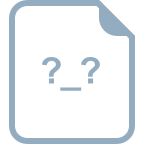














