java 导出excel加序号的注解
时间: 2023-09-04 12:07:15 浏览: 247
你可以使用 Apache POI 库来导出 Excel 文件,并通过自定义注解来添加序号。以下是一个示例代码:
首先,定义一个包含注解的 Java Bean 类,例如:
```java
public class ExcelData {
@ExcelColumn("序号")
private int index;
@ExcelColumn("姓名")
private String name;
@ExcelColumn("年龄")
private int age;
// 省略 getter 和 setter
}
```
其中,`@ExcelColumn` 注解用于标注 Excel 列的标题。
接下来,定义一个 Excel 工具类,例如:
```java
public class ExcelUtils {
public static <T> void export(List<T> dataList, OutputStream outputStream) throws Exception {
Workbook workbook = new XSSFWorkbook();
Sheet sheet = workbook.createSheet("Sheet1");
// 写入表头
Row headerRow = sheet.createRow(0);
Field[] fields = dataList.get(0).getClass().getDeclaredFields();
int columnIndex = 0;
for (Field field : fields) {
ExcelColumn column = field.getAnnotation(ExcelColumn.class);
if (column != null) {
Cell cell = headerRow.createCell(columnIndex);
cell.setCellValue(column.value());
columnIndex++;
}
}
// 写入数据
int rowIndex = 1;
for (T data : dataList) {
Row dataRow = sheet.createRow(rowIndex);
columnIndex = 0;
for (Field field : fields) {
ExcelColumn column = field.getAnnotation(ExcelColumn.class);
if (column != null) {
Cell cell = dataRow.createCell(columnIndex);
if ("序号".equals(column.value())) {
// 添加序号列
cell.setCellValue(rowIndex);
} else {
field.setAccessible(true);
Object value = field.get(data);
if (value != null) {
cell.setCellValue(value.toString());
}
}
columnIndex++;
}
}
rowIndex++;
}
workbook.write(outputStream);
}
}
```
最后,在使用时,可以这样调用:
```java
List<ExcelData> dataList = new ArrayList<>();
// 添加数据到 dataList
ByteArrayOutputStream outputStream = new ByteArrayOutputStream();
ExcelUtils.export(dataList, outputStream);
```
这样就可以导出带有序号的 Excel 文件了。
阅读全文
相关推荐
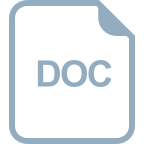
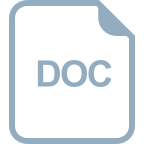
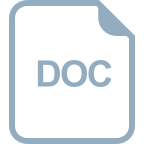
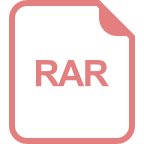

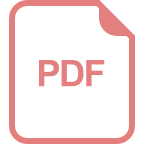
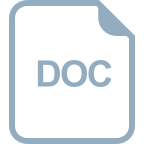
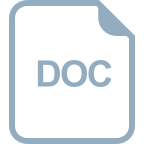










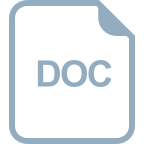