springboot+vue+minIO 秒传,断点续传
时间: 2023-10-05 22:07:17 浏览: 213
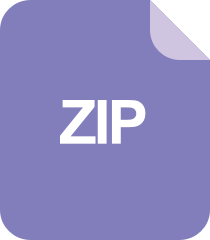
springboot断点续传
要实现Spring Boot和Vue.js的MinIO秒传和断点续传,您可以遵循以下步骤:
1. 创建一个Spring Boot项目并添加MinIO依赖:
```
<dependency>
<groupId>io.minio</groupId>
<artifactId>minio</artifactId>
<version>7.1.0</version>
</dependency>
```
2. 在您的Spring Boot应用程序中配置MinIO。您可以使用`MinioClient`类来进行这个操作。在配置文件中添加以下属性:
```
minio.accessKey=your-access-key
minio.secretKey=your-secret-key
minio.endpoint=your-minio-endpoint
```
3. 在Vue.js中,您可以使用`axios`库来上传文件。以下是上传文件的示例代码:
```
<template>
<div>
<input type="file" v-on:change="uploadFile" />
</div>
</template>
<script>
import axios from 'axios';
export default {
methods: {
async uploadFile(event) {
const file = event.target.files[0];
const url = 'http://localhost:8080/uploadFile';
const data = new FormData();
data.append('file', file);
try {
const response = await axios.post(url, data, {
headers: {
'Content-Type': 'multipart/form-data',
},
});
console.log(response.data);
} catch (error) {
console.log(error);
}
},
},
};
</script>
```
4. 在Spring Boot中,您可以创建一个REST API端点来处理文件上传请求。以下是一个示例代码:
```
@RestController
public class FileUploadController {
@Autowired
private MinioClient minioClient;
@PostMapping("/uploadFile")
public ResponseEntity<String> uploadFile(@RequestParam("file") MultipartFile file) throws Exception {
String fileName = file.getOriginalFilename();
InputStream inputStream = file.getInputStream();
ObjectMetadata metadata = new ObjectMetadata();
metadata.setContentType(file.getContentType());
metadata.setContentLength(file.getSize());
PutObjectOptions options = new PutObjectOptions(metadata);
minioClient.putObject("my-bucket", fileName, inputStream, options);
return ResponseEntity.ok("File uploaded successfully!");
}
}
```
5. 要实现MinIO的断点续传,您可以使用MinIO Java客户端库中提供的`MultiFileUploader`类。以下是一个示例代码:
```
@RestController
public class FileUploadController {
@Autowired
private MinioClient minioClient;
@PostMapping("/uploadFile")
public ResponseEntity<String> uploadFile(@RequestParam("file") MultipartFile file) throws Exception {
String fileName = file.getOriginalFilename();
InputStream inputStream = file.getInputStream();
ObjectMetadata metadata = new ObjectMetadata();
metadata.setContentType(file.getContentType());
metadata.setContentLength(file.getSize());
PutObjectOptions options = new PutObjectOptions(metadata);
MultiFileUploader uploader = new MultiFileUploader(minioClient, "my-bucket", fileName);
uploader.setPartSize(5 * 1024 * 1024); // 5MB
uploader.upload(inputStream, options);
return ResponseEntity.ok("File uploaded successfully!");
}
}
```
以上是Spring Boot和Vue.js的MinIO秒传和断点续传的基本实现。您可以根据自己的需求进行修改和扩展。
阅读全文
相关推荐
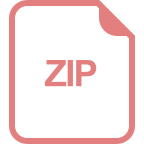
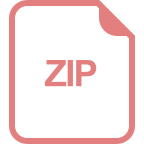
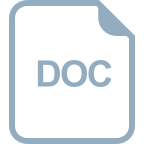
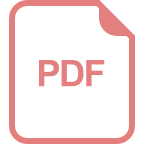
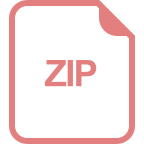
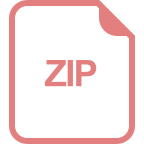
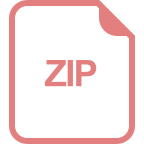
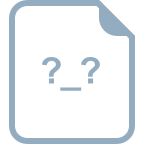
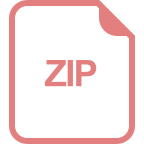
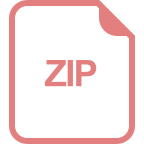
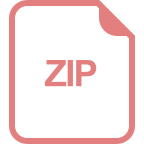
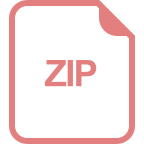