vue路由模式是history,对接微信sdk,可以分享给微信朋友、微信盆友圈,同时别人点击分享的链接还要记录分享次数,这些功能具体实现的步骤,完整代码
时间: 2024-03-04 11:54:10 浏览: 19
实现分享到微信朋友、微信朋友圈以及记录分享次数的功能,可以分为以下几个步骤:
1. 配置微信公众号
在微信公众号后台配置JS-SDK,获取AppID和AppSecret,在开发者中心中配置JS接口安全域名。
2. 引入微信JS-SDK
在HTML文件中引入微信JS-SDK的CDN地址,并在页面加载完成后调用微信JS-SDK的初始化方法:
```javascript
<script src="https://res.wx.qq.com/open/js/jweixin-1.6.0.js"></script>
<script>
wx.config({
debug: false,
appId: 'yourAppId',
timestamp: new Date().getTime(),
nonceStr: 'yourNonceStr',
signature: 'yourSignature',
jsApiList: ['updateAppMessageShareData', 'updateTimelineShareData']
});
wx.ready(function() {
// 微信JS-SDK初始化成功后的回调函数
});
</script>
```
3. 实现分享到微信朋友、微信朋友圈
在初始化成功后,调用微信JS-SDK的`updateAppMessageShareData`和`updateTimelineShareData`方法,分别实现分享到微信朋友和微信朋友圈的功能:
```javascript
wx.ready(function() {
wx.updateAppMessageShareData({
title: '分享标题',
desc: '分享描述',
link: '分享链接',
imgUrl: '分享图标',
success: function() {
// 分享成功后的回调函数
console.log('分享到微信朋友成功');
}
});
wx.updateTimelineShareData({
title: '分享标题',
link: '分享链接',
imgUrl: '分享图标',
success: function() {
// 分享成功后的回调函数
console.log('分享到微信朋友圈成功');
}
});
});
```
4. 记录分享次数
为了记录分享次数,我们可以在分享成功后向后端发送请求,让后端记录分享次数。
```javascript
wx.ready(function() {
wx.updateAppMessageShareData({
title: '分享标题',
desc: '分享描述',
link: '分享链接',
imgUrl: '分享图标',
success: function() {
// 分享成功后的回调函数
console.log('分享到微信朋友成功');
// 向后端发送请求,记录分享次数
axios.post('/share', { type: 'appMessage' }).then(function(res) {
console.log('分享次数已记录');
});
}
});
wx.updateTimelineShareData({
title: '分享标题',
link: '分享链接',
imgUrl: '分享图标',
success: function() {
// 分享成功后的回调函数
console.log('分享到微信朋友圈成功');
// 向后端发送请求,记录分享次数
axios.post('/share', { type: 'timeline' }).then(function(res) {
console.log('分享次数已记录');
});
}
});
});
```
以上代码中,`/share`是向后端发送记录分享次数请求的接口地址,`type`是分享类型,可以是`appMessage`或`timeline`。后端接收到请求后,根据分享类型记录分享次数即可。
完整代码示例:
```javascript
<script src="https://res.wx.qq.com/open/js/jweixin-1.6.0.js"></script>
<script>
wx.config({
debug: false,
appId: 'yourAppId',
timestamp: new Date().getTime(),
nonceStr: 'yourNonceStr',
signature: 'yourSignature',
jsApiList: ['updateAppMessageShareData', 'updateTimelineShareData']
});
wx.ready(function() {
wx.updateAppMessageShareData({
title: '分享标题',
desc: '分享描述',
link: '分享链接',
imgUrl: '分享图标',
success: function() {
// 分享成功后的回调函数
console.log('分享到微信朋友成功');
// 向后端发送请求,记录分享次数
axios.post('/share', { type: 'appMessage' }).then(function(res) {
console.log('分享次数已记录');
});
}
});
wx.updateTimelineShareData({
title: '分享标题',
link: '分享链接',
imgUrl: '分享图标',
success: function() {
// 分享成功后的回调函数
console.log('分享到微信朋友圈成功');
// 向后端发送请求,记录分享次数
axios.post('/share', { type: 'timeline' }).then(function(res) {
console.log('分享次数已记录');
});
}
});
});
</script>
```
相关推荐
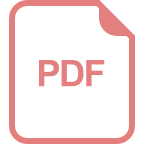
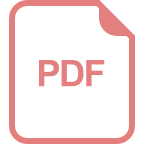
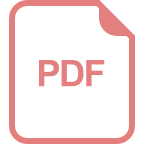














