java中rep在python中的表达式.
时间: 2023-02-08 07:09:39 浏览: 151
在 Python 中,可以使用正则表达式模块 `re` 中的 `sub()` 函数来实现和 Java 中 `replace()` 函数相似的功能。
语法: re.sub(pattern, repl, string, count=0, flags=0)
参数:
- pattern: 正则表达式模式
- repl: 替换文本
- string: 待处理字符串
- count: 匹配个数
- flags: 可选标志
例如:
```
import re
string = "Hello, Java and Python"
new_string = re.sub("Java", "Python", string)
print(new_string)
```
输出: "Hello, Python and Python"
这里将原字符串中的 Java 替换为 Python
相关问题
java中rep在python中的表达式
### 回答1:
在 Python 中,用正则表达式(re)库来替代 Java 中的 `replace()` 方法。使用正则表达式的 `sub()` 方法可以替换字符串中符合条件的部分。示例代码如下:
```
import re
original_string = "this is the original string"
pattern = "original"
replacement = "new"
new_string = re.sub(pattern, replacement, original_string)
print(new_string) # Output: this is the new string
```
其中,`re.sub(pattern, replacement, original_string)`表示把original_string中符合pattern的部分替换成replacement。
### 回答2:
在Python中,我们可以使用replace()方法来替换字符串中的某个部分,而在Java中,我们可以使用replaceAll()或replaceFirst()方法来实现类似的功能。
在Python中,replace()方法的语法如下:
new_string = string.replace(old_value, new_value)
其中,string是原始字符串,old_value是需要被替换的部分,new_value是新的字符串。
而在Java中,replaceAll()方法的语法如下:
String newString = originalString.replaceAll(oldValue, newValue);
或者使用replaceFirst()方法:
String newString = originalString.replaceFirst(oldValue, newValue);
这两个方法都是用来替换字符串中的某个部分。其中,originalString是原始字符串,oldValue是需要被替换的部分,newValue是新的字符串。
需要注意的是,在Java中,replaceAll()方法可以使用正则表达式进行匹配,而replace()方法则只能替换字符串中的具体值。
总结来说,Python中的rep可以用replace()方法来实现,在Java中则可以使用replaceAll()或replaceFirst()方法。
### 回答3:
在Java中,"rep"这个词通常表示的是代表"repetition"(重复)的缩写,而在Python中的对应表达式是" * "。它可以用于字符串、列表、元组和其他可迭代对象,用于生成一个重复指定次数的新的对象。下面是一些示例:
1. 字符串重复:
```python
string = "Hello"
repeated_string = string * 3
print(repeated_string)
# 输出:HelloHelloHello
```
2. 列表重复:
```python
list1 = [1, 2, 3]
repeated_list = list1 * 2
print(repeated_list)
# 输出:[1, 2, 3, 1, 2, 3]
```
3. 元组重复:
```python
tuple1 = (1, 2, 3)
repeated_tuple = tuple1 * 4
print(repeated_tuple)
# 输出:(1, 2, 3, 1, 2, 3, 1, 2, 3, 1, 2, 3)
```
通过使用" * "运算符,我们可以简便地在Python中实现对字符串、列表和元组的重复操作,这与Java中的"rep"表示的功能类似。
npar = size(REP.pos_fit,1); REP.grid_idx = zeros(npar,1); REP.grid_subidx = zeros(npar,ndim); for n = 1:1:npar idnames = []; for d = 1:1:ndim % REP.grid_subidx(n,d) = find(REP.pos_fit(n,d)<=REP.hypercube_limits(:,d)',1,'first')-1; REP.grid_subidx(n,d) = find(REP.pos_fit(n,d)<=REP.hypercube_limits(:,d)',1,'first')-1; if(REP.grid_subidx(n,d)==0), REP.grid_subidx(n,d) = 1; end idnames = [idnames ',' num2str(REP.grid_subidx(n,d))]; end REP.grid_idx(n) = eval(['sub2ind(ngrid.*ones(1,ndim)' idnames ');']); end
这段代码用于将存储库中每个解的位置映射到对应的网格索引。
1. 首先,获取存储库中适应度矩阵的行数,即解的数量,并保存在变量 `npar` 中。
2. 创建大小为 `(npar × 1)` 的零向量 `REP.grid_idx`,用于存储每个解所在的网格索引。
3. 创建大小为 `(npar × ndim)` 的零矩阵 `REP.grid_subidx`,用于存储每个解在每个维度上的子索引。
4. 使用两个嵌套的循环,对每个解进行处理。
- 外部循环 `n` 遍历解的索引,从1到 `npar`。
- 内部循环 `d` 遍历每个维度,从1到 `ndim`。
5. 在内部循环中,使用 `find` 函数找到每个解在当前维度上满足小于等于超立方体边界限制的第一个位置,并将结果减去1,保存在 `REP.grid_subidx(n,d)` 中。这样可以得到每个解在每个维度上的子索引。
6. 如果 `REP.grid_subidx(n,d)` 的值为0,将其设置为1。这是为了处理那些处于超立方体边界的解。
7. 构建一个字符串 `idnames`,用于构造 `sub2ind` 函数的输入参数。该字符串包含每个维度上的子索引,用逗号分隔。
8. 调用 `eval` 函数计算 `sub2ind` 函数的输入参数,并将结果赋值给 `REP.grid_idx(n)`,即当前解所在的网格索引。
这段代码的目的是将存储库中每个解的位置映射到对应的网格索引,并将结果存储在 `REP.grid_idx` 和 `REP.grid_subidx` 中。这样可以根据网格索引来查找和操作存储库中的解。
阅读全文
相关推荐
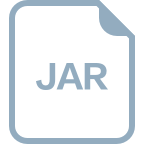
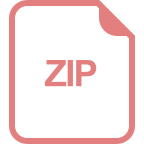
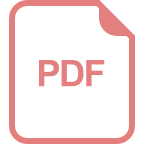
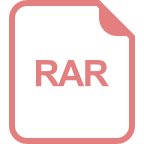
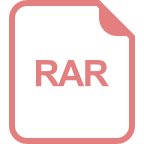
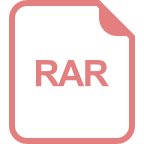
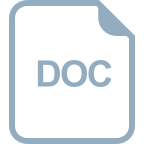
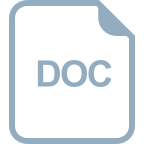





