java使用Poi-tl,如何让网络图片保持比例不变
时间: 2024-04-28 10:19:08 浏览: 12
在使用Poi-tl插入网络图片时,我们可以设置图片的宽度和高度来控制图片的大小,但是要保持图片的比例不变,我们需要按照以下步骤进行操作:
1. 获取图片的实际宽度和高度。
可以使用Java的ImageIO类的read()方法来读取网络图片,然后使用getWidth()和getHeight()方法获取图片的实际宽度和高度。
2. 根据图片的实际宽度和高度,计算出图片的缩放比例。
我们可以根据需要显示图片的宽度或高度和实际宽度或高度的比例来计算出图片的缩放比例,然后将其应用于图片的宽度或高度,以保持图片的比例不变。
3. 将缩放后的图片插入到文档中。
使用Poi-tl插入图片时,可以使用setWidth()和setHeight()方法来设置图片的宽度和高度,将计算出的缩放比例应用于其中一个参数即可。
下面是一个示例代码:
```java
import java.awt.image.BufferedImage;
import java.io.IOException;
import java.net.URL;
import javax.imageio.ImageIO;
import org.apache.poi.util.IOUtils;
import org.apache.poi.xwpf.usermodel.XWPFDocument;
import org.apache.poi.xwpf.usermodel.XWPFPicture;
import org.apache.poi.xwpf.usermodel.XWPFRun;
import org.openxmlformats.schemas.drawingml.x2006.main.CTNonVisualDrawingProps;
import org.openxmlformats.schemas.drawingml.x2006.main.CTPositiveSize2D;
import org.openxmlformats.schemas.drawingml.x2006.main.CTRegularTextRun;
import org.openxmlformats.schemas.drawingml.x2006.main.CTShapeProperties;
import org.openxmlformats.schemas.drawingml.x2006.main.CTTransform2D;
import org.openxmlformats.schemas.drawingml.x2006.main.STPositiveCoordinate;
public class InsertImageDemo {
public static void main(String[] args) throws Exception {
XWPFDocument document = new XWPFDocument();
XWPFRun run = document.createParagraph().createRun();
String imageUrl = "https://example.com/image.png";
BufferedImage image = readImageFromUrl(imageUrl);
int imageWidth = image.getWidth();
int imageHeight = image.getHeight();
int maxWidth = 500; // 最大宽度
int maxHeight = 500; // 最大高度
double widthRatio = (double) maxWidth / imageWidth;
double heightRatio = (double) maxHeight / imageHeight;
double ratio = Math.min(widthRatio, heightRatio); // 缩放比例
int width = (int) (imageWidth * ratio);
int height = (int) (imageHeight * ratio);
byte[] imageData = readImageDataFromUrl(imageUrl);
XWPFPicture picture = run.addPicture(IOUtils.toByteArray(imageData), XWPFDocument.PICTURE_TYPE_PNG, "image.png", width, height);
CTTransform2D transform = picture.getCTPicture().getSpPr().getXfrm();
CTPositiveSize2D extent = transform.getExt();
extent.setCx(BigInteger.valueOf(width * 9525));
extent.setCy(BigInteger.valueOf(height * 9525));
CTNonVisualDrawingProps nvdp = picture.getCTPicture().getNvPicPr().getNvNonVisualDrawingProps();
nvdp.setName("image.png");
nvdp.setId(BigInteger.valueOf(1));
CTRegularTextRun r = picture.getCTPicture().getNvPicPr().getCNvPicPr().createR();
r.setT("");
CTShapeProperties sp = picture.getCTPicture().getSpPr();
sp.setBw(0);
sp.setBwMode(STStrokeLineStyle.NONE);
sp.setNoFill(null);
sp.setLn(null);
document.write(new FileOutputStream("output.docx"));
}
private static BufferedImage readImageFromUrl(String imageUrl) throws IOException {
URL url = new URL(imageUrl);
return ImageIO.read(url);
}
private static byte[] readImageDataFromUrl(String imageUrl) throws IOException {
URL url = new URL(imageUrl);
InputStream is = url.openStream();
ByteArrayOutputStream baos = new ByteArrayOutputStream();
byte[] buffer = new byte[1024];
int length;
while ((length = is.read(buffer)) != -1) {
baos.write(buffer, 0, length);
}
is.close();
return baos.toByteArray();
}
}
```
在上面的代码中,我们使用ImageIO类读取网络图片,并使用setWidth()和setHeight()方法设置图片的宽度和高度,然后使用CTPositiveSize2D类设置图片的大小,最后将图片插入到文档中。
相关推荐
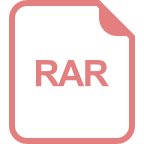
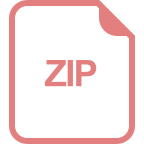














