使用C++在VS2019中制作一个GUI来展示倒计时的完整代码
时间: 2023-06-26 18:08:09 浏览: 83
以下是使用C++和MFC库在VS2019中制作倒计时GUI的完整代码:
```
// CountdownTimerDlg.h
#pragma once
#include "afxwin.h"
class CCountdownTimerDlg : public CDialogEx
{
public:
CCountdownTimerDlg(CWnd* pParent = nullptr); // standard constructor
virtual ~CCountdownTimerDlg();
// Dialog Data
#ifdef AFX_DESIGN_TIME
enum { IDD = IDD_COUNTDOWN_TIMER_DIALOG };
#endif
protected:
virtual void DoDataExchange(CDataExchange* pDX); // DDX/DDV support
DECLARE_MESSAGE_MAP()
private:
int m_hours;
int m_minutes;
int m_seconds;
CStatic m_display;
bool m_timer_running;
UINT_PTR m_timer_id;
void UpdateDisplay();
public:
virtual BOOL OnInitDialog();
afx_msg void OnBnClickedStartButton();
afx_msg void OnBnClickedStopButton();
afx_msg void OnTimer(UINT_PTR nIDEvent);
};
// CountdownTimerDlg.cpp
#include "stdafx.h"
#include "CountdownTimer.h"
#include "CountdownTimerDlg.h"
#include "afxdialogex.h"
#ifdef _DEBUG
#define new DEBUG_NEW
#endif
// CCountdownTimerDlg dialog
CCountdownTimerDlg::CCountdownTimerDlg(CWnd* pParent /*=nullptr*/)
: CDialogEx(IDD_COUNTDOWN_TIMER_DIALOG, pParent),
m_hours(0), m_minutes(0), m_seconds(0), m_timer_running(false), m_timer_id(0)
{
}
CCountdownTimerDlg::~CCountdownTimerDlg()
{
}
void CCountdownTimerDlg::DoDataExchange(CDataExchange* pDX)
{
CDialogEx::DoDataExchange(pDX);
DDX_Control(pDX, IDC_DISPLAY, m_display);
}
BEGIN_MESSAGE_MAP(CCountdownTimerDlg, CDialogEx)
ON_BN_CLICKED(IDC_START_BUTTON, &CCountdownTimerDlg::OnBnClickedStartButton)
ON_BN_CLICKED(IDC_STOP_BUTTON, &CCountdownTimerDlg::OnBnClickedStopButton)
ON_WM_TIMER()
END_MESSAGE_MAP()
// CCountdownTimerDlg message handlers
BOOL CCountdownTimerDlg::OnInitDialog()
{
CDialogEx::OnInitDialog();
// Set the timer interval to 1 second
SetTimer(m_timer_id, 1000, NULL);
return TRUE;
}
void CCountdownTimerDlg::OnBnClickedStartButton()
{
// Get the user input for hours, minutes, and seconds
m_hours = GetDlgItemInt(IDC_HOURS_EDIT);
m_minutes = GetDlgItemInt(IDC_MINUTES_EDIT);
m_seconds = GetDlgItemInt(IDC_SECONDS_EDIT);
// Start the timer
m_timer_running = true;
}
void CCountdownTimerDlg::OnBnClickedStopButton()
{
// Stop the timer
m_timer_running = false;
}
void CCountdownTimerDlg::OnTimer(UINT_PTR nIDEvent)
{
if (m_timer_running)
{
// Decrement the remaining time
if (m_seconds > 0)
{
m_seconds--;
}
else if (m_minutes > 0)
{
m_minutes--;
m_seconds = 59;
}
else if (m_hours > 0)
{
m_hours--;
m_minutes = 59;
m_seconds = 59;
}
else
{
// Time's up!
m_timer_running = false;
}
UpdateDisplay();
}
CDialogEx::OnTimer(nIDEvent);
}
void CCountdownTimerDlg::UpdateDisplay()
{
CString display_text;
display_text.Format(_T("%02d:%02d:%02d"), m_hours, m_minutes, m_seconds);
m_display.SetWindowText(display_text);
}
```
在这个代码中,我们创建了一个名为CCountdownTimerDlg的对话框类。在OnInitDialog函数中,我们设置了定时器间隔为1秒,并在OnTimer函数中处理了倒计时逻辑。用户通过在编辑框中输入小时、分钟、秒数来指定倒计时时间,并通过点击开始和停止按钮来启动和停止倒计时。最后,我们在UpdateDisplay函数中更新了GUI中的显示内容。
阅读全文
相关推荐









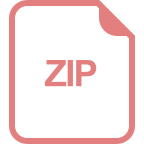








