Write a python script to solve the following problem:Given an input string s and a pattern p, implement regular expression matching with support for '.' and '*' where: '.' Matches any single character. '*' Matches zero or more of the preceding element. The matching should cover the entire input string (not partial).
时间: 2023-02-09 10:59:28 浏览: 201
在 Python 中实现正则表达式匹配有很多现成的库可以使用,例如 `re` 模块。但是,这里我们可以手动实现一个简单的匹配函数:
```python
def match(s: str, p: str) -> bool:
# 如果模式串为空,则只有当输入串也为空时才匹配成功
if not p:
return not s
# 如果模式串不为空,则分类讨论
# 第一个字符是否是 *
if len(p) > 1 and p[1] == '*':
# 如果第一个字符是 .,则匹配任意一个字符(包括空串)
if p[0] == '.':
return match(s, p[2:]) or match(s[1:], p)
# 否则,匹配任意多个 p[0]
else:
i = 0
while i < len(s) and s[i] == p[0]:
i += 1
return match(s[i:], p[2:])
# 如果第一个字符不是 *,则判断是否匹配
else:
if not s:
return False
if p[0] == '.' or s[0] == p[0]:
return match(s[1:], p[1:])
else:
return False
```
这个函数实现了对 . 和 * 的支持,使用递归的方式实现了匹配过程。
举个例子:
```python
assert match("aa", "a") == False
assert match("aa", "aa") == True
assert match("abc", "a.c") == True
assert match("abbb", "ab*") == True
assert match("ac", "ab*c") == True
```
希望这个函数能帮助你解决问题。
阅读全文
相关推荐
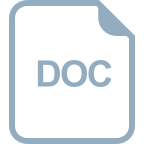
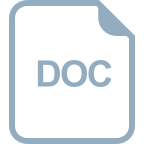
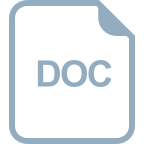
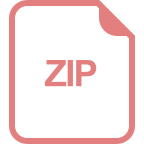
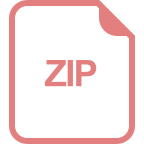
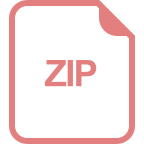
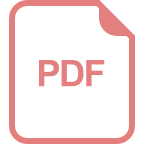
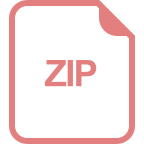
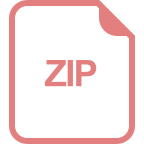
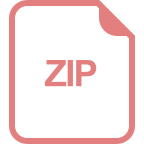
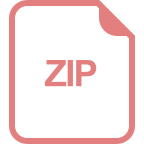
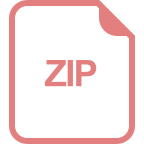
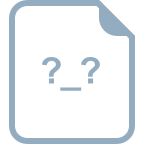
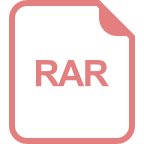
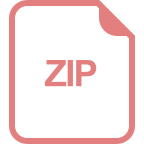
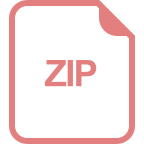
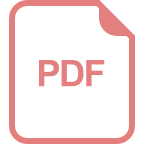
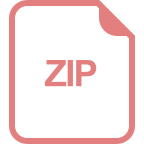