thymeleaf访问数据库代码
时间: 2023-10-14 11:18:36 浏览: 78
Thymeleaf 是一个用于Web和独立环境的现代服务器端Java模板引擎。它能够处理HTML,XML,JavaScript,CSS等文件。它可以集成Spring框架,提供了一个非常方便的方式来访问数据库。下面是一个使用Thymeleaf访问数据库的简单示例:
在pom.xml中添加以下依赖:
```xml
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-thymeleaf</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-jdbc</artifactId>
</dependency>
```
在application.properties中配置数据库:
```
spring.datasource.url=jdbc:mysql://localhost:3306/test
spring.datasource.username=root
spring.datasource.password=root
spring.datasource.driver-class-name=com.mysql.jdbc.Driver
```
在Java代码中使用JdbcTemplate访问数据库:
```java
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.jdbc.core.JdbcTemplate;
import org.springframework.stereotype.Component;
import java.util.List;
@Component
public class UserRepository {
@Autowired
private JdbcTemplate jdbcTemplate;
public List<User> getUsers() {
return jdbcTemplate.query(
"SELECT id, name, email FROM users",
(rs, rowNum) -> new User(
rs.getLong("id"),
rs.getString("name"),
rs.getString("email")
)
);
}
}
```
在Thymeleaf模板中使用UserRepository获取数据:
```html
<!DOCTYPE html>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<title>Users</title>
</head>
<body>
<h1>Users</h1>
<table>
<thead>
<tr>
<th>ID</th>
<th>Name</th>
<th>Email</th>
</tr>
</thead>
<tbody>
<tr th:each="user : ${userRepository.getUsers()}">
<td th:text="${user.id}"></td>
<td th:text="${user.name}"></td>
<td th:text="${user.email}"></td>
</tr>
</tbody>
</table>
</body>
</html>
```
在上面的示例中,我们创建了一个UserRepository类,它使用JdbcTemplate来查询数据库中的用户数据。然后,在Thymeleaf模板中使用UserRepository来获取数据,并在表格中显示它们。
阅读全文
相关推荐
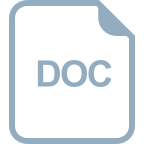
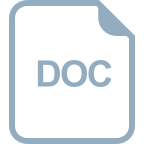
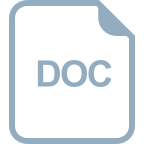
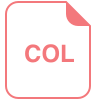
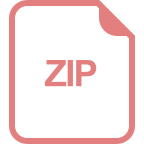
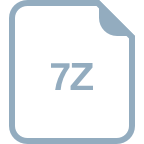
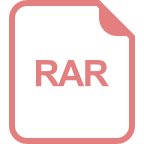
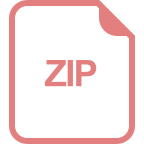
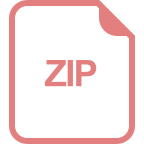
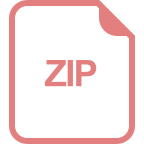
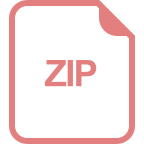
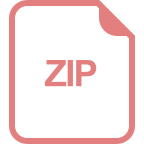
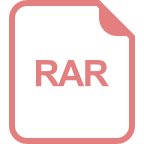
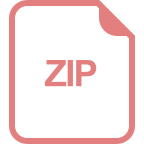
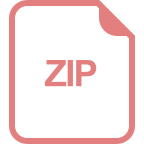
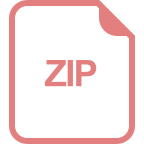
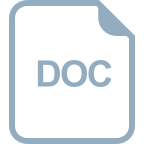
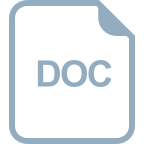
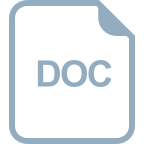