springboot 读取文件夹返回树给前端
时间: 2023-11-09 22:49:54 浏览: 40
要实现这个功能,可以使用SpringBoot提供的自动配置来读取文件夹中的所有文件和文件夹,并将它们组织成树形结构。然后将该结构转换为JSON格式返回给前端。
以下是实现步骤:
1. 添加依赖
在项目的pom.xml文件中添加以下依赖:
```xml
<dependency>
<groupId>com.fasterxml.jackson.core</groupId>
<artifactId>jackson-databind</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
```
2. 创建实体类
创建一个名为`FileNode`的实体类,用于存储文件和文件夹的信息:
```java
public class FileNode {
private String name;
private String path;
private boolean isFile;
private List<FileNode> children;
// getter and setter methods
}
```
其中,`name`表示文件或文件夹的名称,`path`表示文件或文件夹的路径,`isFile`表示是否为文件,`children`表示当前节点的子节点列表。
3. 创建控制器
创建一个名为`FileController`的控制器,用于处理前端请求:
```java
@RestController
@RequestMapping("/file")
public class FileController {
@GetMapping("/tree")
public FileNode getFilesTree() {
String rootPath = "/path/to/your/folder";
return getFileNode(new File(rootPath));
}
private FileNode getFileNode(File file) {
FileNode node = new FileNode();
node.setName(file.getName());
node.setPath(file.getPath());
node.setFile(file.isFile());
if (file.isDirectory()) {
File[] children = file.listFiles();
if (children != null) {
List<FileNode> childNodes = new ArrayList<>();
for (File child : children) {
childNodes.add(getFileNode(child));
}
node.setChildren(childNodes);
}
}
return node;
}
}
```
上述代码中,`getFilesTree()`方法用于处理前端请求,返回整个文件夹的树形结构。`getFileNode()`方法则用于递归构建每个文件夹节点的子节点列表。
4. 将结构转换为JSON格式
由于前端需要的是JSON格式的数据,因此需要将`FileNode`实体类转换为JSON格式。可以使用Jackson库来完成这个转换:
```java
public class JsonUtils {
private static final ObjectMapper objectMapper = new ObjectMapper();
public static String toJson(Object obj) {
try {
return objectMapper.writeValueAsString(obj);
} catch (JsonProcessingException e) {
throw new RuntimeException(e);
}
}
}
```
然后在`FileController`中调用`JsonUtils.toJson()`方法将`FileNode`实体类转换为JSON格式:
```java
@RestController
@RequestMapping("/file")
public class FileController {
@GetMapping(value = "/tree", produces = "application/json")
public String getFilesTree() {
String rootPath = "/path/to/your/folder";
FileNode rootNode = getFileNode(new File(rootPath));
return JsonUtils.toJson(rootNode);
}
// getFileNode() method is the same as before
}
```
5. 测试
现在可以启动应用程序并在浏览器中访问`http://localhost:8080/file/tree`来获取整个文件夹的树形结构。
相关推荐
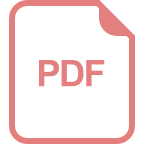










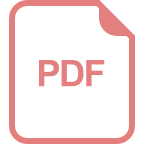
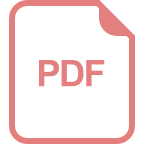
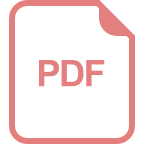
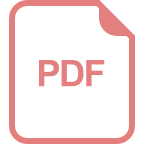
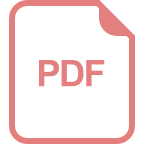