3.统计某本小说中人物出场次数,并形成词云图。(至少统计出出场次数最多的前5个人物;词云图与统计的人物次数基本一致)
时间: 2024-01-30 15:06:39 浏览: 46
这个问题比较复杂,需要分多个步骤来实现。
首先,我们需要读取小说文本,并使用 jieba 分词库进行分词。假设小说文本保存在 novel.txt 文件中,可以使用以下代码实现:
```python
import jieba
# 读取小说文本
with open("novel.txt", "r", encoding="utf-8") as f:
content = f.read()
# 对文本进行分词
words = jieba.lcut(content)
```
接着,我们需要统计人物出现次数。可以使用 Python 的字典来进行统计。具体代码如下:
```python
# 统计人物出现次数
person_dict = {}
for i in range(len(words) - 1):
if words[i] == "某" and words[i+1] == "人":
person = words[i-1]
if person in person_dict:
person_dict[person] += 1
else:
person_dict[person] = 1
```
在以上代码中,我们首先定义了一个空字典 person_dict,用于存储人物出现次数。然后遍历分词后的词语列表,如果当前词语为“人”,前一个词语为“某”,则认为这是一个人物出现的位置。我们将其前一个词语作为人物名称,然后在字典中进行统计。
接着,我们需要将统计结果按照出现次数从大到小排序,并只取前 5 个人物。可以使用以下代码实现:
```python
# 按照出现次数进行排序
person_list = sorted(person_dict.items(), key=lambda x: x[1], reverse=True)
# 取出前 5 个人物
top_person_list = person_list[:5]
```
在以上代码中,我们使用了 Python 的 sorted 函数,按照字典中的值(即出现次数)进行排序。然后使用切片操作取出前 5 个人物。
最后,我们需要使用词云库来生成词云图。可以使用 wordcloud 库来实现。具体代码如下:
```python
import matplotlib.pyplot as plt
from wordcloud import WordCloud
# 生成词云图
wordcloud = WordCloud(font_path="msyh.ttc", background_color="white")
person_dict = dict(top_person_list)
wordcloud.generate_from_frequencies(person_dict)
# 显示词云图
plt.imshow(wordcloud, interpolation="bilinear")
plt.axis("off")
plt.show()
```
在以上代码中,我们首先引入了 matplotlib.pyplot 和 wordcloud 两个库。然后创建了一个 WordCloud 对象,并使用 generate_from_frequencies 方法生成词云图。最后使用 imshow 方法将词云图显示出来。
完整代码如下:
```python
import jieba
import matplotlib.pyplot as plt
from wordcloud import WordCloud
# 读取小说文本
with open("novel.txt", "r", encoding="utf-8") as f:
content = f.read()
# 对文本进行分词
words = jieba.lcut(content)
# 统计人物出现次数
person_dict = {}
for i in range(len(words) - 1):
if words[i] == "某" and words[i+1] == "人":
person = words[i-1]
if person in person_dict:
person_dict[person] += 1
else:
person_dict[person] = 1
# 按照出现次数进行排序
person_list = sorted(person_dict.items(), key=lambda x: x[1], reverse=True)
# 取出前 5 个人物
top_person_list = person_list[:5]
# 生成词云图
wordcloud = WordCloud(font_path="msyh.ttc", background_color="white")
person_dict = dict(top_person_list)
wordcloud.generate_from_frequencies(person_dict)
# 显示词云图
plt.imshow(wordcloud, interpolation="bilinear")
plt.axis("off")
plt.show()
```
需要注意的是,词云图的生成需要安装 wordcloud 库,可以使用 `pip install wordcloud` 命令进行安装。
相关推荐
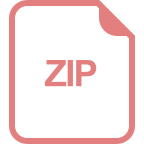








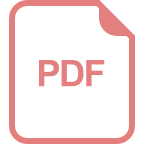
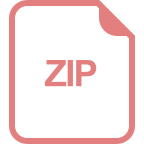