图片中的数字识别训练模块代码
时间: 2024-01-16 16:05:39 浏览: 30
好的,图片中的数字识别训练模块代码可以使用Python编写。以下是一个基于Keras库的图片中的数字识别训练模块的示例代码:
```
import tensorflow as tf
from tensorflow import keras
import numpy as np
import cv2
# 加载数据集
(x_train, y_train), (x_test, y_test) = keras.datasets.mnist.load_data()
# 数据预处理
x_train = x_train.reshape(x_train.shape[0], 28, 28, 1).astype('float32') / 255
x_test = x_test.reshape(x_test.shape[0], 28, 28, 1).astype('float32') / 255
y_train = keras.utils.to_categorical(y_train, 10)
y_test = keras.utils.to_categorical(y_test, 10)
# 定义模型
model = keras.Sequential([
keras.layers.Conv2D(32, (3, 3), activation='relu', input_shape=(28, 28, 1)),
keras.layers.MaxPooling2D((2, 2)),
keras.layers.Flatten(),
keras.layers.Dense(10, activation='softmax')
])
# 编译模型
model.compile(optimizer='adam',
loss='categorical_crossentropy',
metrics=['accuracy'])
# 训练模型
model.fit(x_train, y_train, epochs=5, validation_data=(x_test, y_test))
# 保存模型
model.save('mnist_model.h5')
# 加载模型
model = keras.models.load_model('mnist_model.h5')
# 读取待识别图片
img = cv2.imread('test_img.png', cv2.IMREAD_GRAYSCALE)
img = cv2.resize(img, (28, 28))
img = np.reshape(img, [1, 28, 28, 1])
img = img.astype('float32') / 255
# 进行识别
result = model.predict(img)
digit = np.argmax(result)
print('The digit is:', digit)
```
这段代码的主要过程如下:
1. 加载MNIST数据集并进行数据预处理
2. 定义卷积神经网络模型并编译模型
3. 训练模型并保存训练好的模型
4. 加载训练好的模型并读取待识别的图片
5. 对待识别的图片进行预处理,并使用训练好的模型进行识别
6. 输出识别结果
其中,待识别的图片需要以灰度图像的形式读入,并且需要将其调整为28x28的大小。最后,通过`argmax`函数获取识别结果。
相关推荐
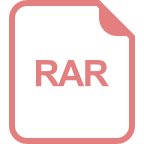
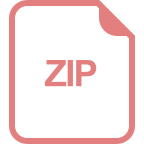
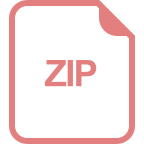














