char str[] = "Hello"; char *ptr ;ptr= str;
时间: 2024-04-08 10:30:24 浏览: 123
在你提供的代码中,首先定义了一个字符数组 `str` 并初始化为 "Hello":
```c
char str[] = "Hello";
```
然后,声明了一个字符指针 `ptr`:
```c
char *ptr;
```
接下来,通过赋值操作将字符数组 `str` 的地址赋给了指针 `ptr`:
```c
ptr = str;
```
这样,指针 `ptr` 指向了字符数组 `str` 的第一个元素。
注意,这里的赋值操作是将数组的地址赋给指针,而不是通过字符串常量的赋值。这意味着指针 `ptr` 现在指向了字符数组 `str`,你可以通过指针 `ptr` 来访问和操作字符数组中的内容。这种赋值操作是有效的,并且可以使用指针 `ptr` 来访问和修改字符数组 `str` 中的数据。
相关问题
#include <stdio.h> void to_upper(char *str){char *ptr = str; while (*ptr != 'lO'){ if (*ptr >= 'a' && *ptr <= 'z'){*ptr += 'A' - 'a'; } ptr++;}} int main() { char str[= "Hello, World!";to_upper(str); printf("%s",str);return o; }
这段代码有一些错误,无法编译通过。首先是char str[= "Hello, World!";这个定义数组的语句中,等号后面应该是数组长度,而不是一个等于号。另外,在函数to_upper中,应该是*ptr != 'O',而不是*ptr != 'lO'。最后,在循环内部修改字符的时候,应该是*ptr += 'A' - 'a',而不是*ptr += 'A' - 'a'; 。修改后的代码如下:
```
#include <stdio.h>
void to_upper(char *str){
char *ptr = str;
while (*ptr != 'O'){
if (*ptr >= 'a' && *ptr <= 'z'){
*ptr += 'A' - 'a';
}
ptr++;
}
}
int main() {
char str[] = "Hello, World!";
to_upper(str);
printf("%s",str);
return 0;
}
```
这段代码的功能是将字符串中的小写字母转换为大写字母,直到遇到字符'O'为止。输出结果为"HELL, WRLD!"。
#include <stdio.h> void to_upper(char *str) { char *ptr = str; while (*ptr != '\0') { if (*ptr >= 'a' && *ptr <= 'z') { *ptr += 'A' - 'a'; } ptr++; } } int main() { char str[]= "Hello, World!";to_upper(str); printf("%s",str);return 0; }
这段代码是一个将字符串中的小写字母转换成大写字母的程序。函数to_upper接受一个指向字符串的指针,然后遍历字符串中的每个字符,如果当前字符是小写字母,则将其转换为大写字母。最后在主函数中,定义一个字符串数组str并初始化为"Hello, World!",然后调用to_upper函数将其中的小写字母转换为大写字母,并输出结果。
需要注意的是,在遍历字符串的过程中,当指针ptr指向字符串末尾'\0'时,循环结束。另外,在修改字符的时候,应该是*ptr += 'A' - 'a',而不是*ptr += 'A' - 'a';。
修改后的代码如下:
```
#include <stdio.h>
void to_upper(char *str) {
char *ptr = str;
while (*ptr != '\0') {
if (*ptr >= 'a' && *ptr <= 'z') {
*ptr += 'A' - 'a';
}
ptr++;
}
}
int main() {
char str[]= "Hello, World!";
to_upper(str);
printf("%s",str);
return 0;
}
```
输出结果为"HELLO, WORLD!"。
阅读全文
相关推荐
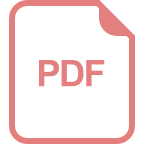













