@Service public class CateService extends ServiceImpl<CateMapper, Cate> { @Resource private CateMapper cateMapper; public List<Cate> listCate(Cate cate) { return cateMapper.selectList(Wrappers.<Cate>lambdaQuery() .like(StringUtils.isNotBlank(cate.getName()), Cate::getName, cate.getName()) .eq(cate.getActive() != null, Cate::getActive, cate.getActive()) .orderByAsc(Cate::getSort) ); } }
时间: 2024-04-15 18:24:45 浏览: 116
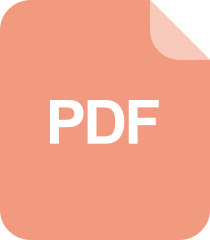
@Validated验证List集合的方法示例
这是一个名为 CateService 的服务类,在 Spring 框架中使用 @Service 注解进行标识。它继承自 ServiceImpl<CateMapper, Cate>,表示它是一个通用的 CRUD 服务类,针对 Cate 实体类的数据库操作。
在该类中,使用 @Resource 注解对 CateMapper 进行注入,以便在方法中使用该对象进行数据库操作。
listCate 方法用于查询符合条件的 Cate 对象列表。它通过 cateMapper 对象调用 selectList 方法,并利用 MyBatis-Plus 提供的 Wrappers 工具类构建查询条件。具体的查询条件包括:
- 使用 lambdaQuery 方法创建查询对象
- 使用 like 方法进行模糊查询,如果 cate.getName() 不为空,则根据 Cate 实体类的 name 字段进行模糊匹配
- 使用 eq 方法进行相等查询,如果 cate.getActive() 不为 null,则根据 Cate 实体类的 active 字段进行相等匹配
- 使用 orderByAsc 方法按照 Cate 实体类的 sort 字段进行升序排序
最后,返回符合条件的 Cate 对象列表。
需要注意的是,CateService 类通过继承 ServiceImpl 类获得了一些通用的数据库操作方法,例如 save、update、remove 等。同时,通过 @Service 注解,Spring 会将该类作为一个服务类进行管理,可以通过依赖注入的方式在其他地方使用该服务类的实例。
阅读全文
相关推荐
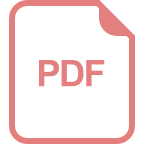
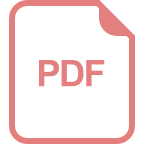


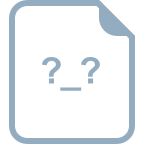
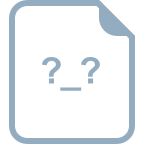
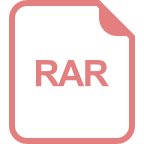
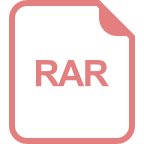
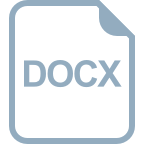
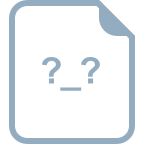
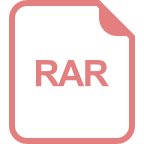
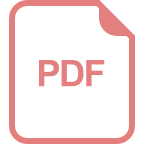
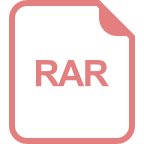
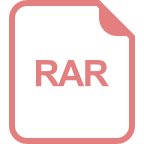
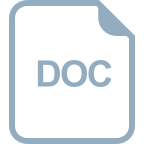
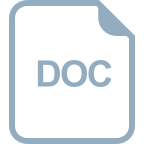
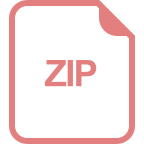
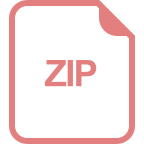