python爬虫爬取今日头条网页数据完整代码
时间: 2023-07-26 18:04:56 浏览: 86
好的,以下是完整的代码:
```python
import requests
from bs4 import BeautifulSoup
url = 'https://www.toutiao.com/ch/news_hot/'
headers = {
'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/58.0.3029.110 Safari/537.3'}
response = requests.get(url, headers=headers)
html = response.text
soup = BeautifulSoup(html, 'html.parser')
titles = soup.find_all('div', {'class': 'title-box'})
for title in titles:
print(title.a.string)
```
在这个示例中,我们设置了请求头,模拟浏览器发送请求,避免被反爬机制拦截。同时使用了BeautifulSoup库解析网页源代码,并提取了新闻标题。
当然,如果要爬取更多的数据,可以使用Selenium库模拟浏览器操作,或者使用API接口等方式获取数据。
相关问题
python爬虫爬取今日头条网页数据
要爬取今日头条网页数据,可以使用Python的requests库和BeautifulSoup库。
首先,使用requests库发送GET请求获取网页源代码:
```python
import requests
url = 'https://www.toutiao.com/ch/news_hot/'
response = requests.get(url)
html = response.text
```
接着,使用BeautifulSoup库解析网页源代码,并提取需要的数据:
```python
from bs4 import BeautifulSoup
soup = BeautifulSoup(html, 'html.parser')
titles = soup.find_all('div', {'class': 'title-box'})
for title in titles:
print(title.a.string)
```
上面的代码中,通过`find_all`方法匹配所有class为`title-box`的`div`元素,然后遍历每个元素,提取其中的`a`标签的文本内容,即为新闻标题。
当然,这只是一个简单的示例,实际爬取数据需要考虑到反爬机制和数据清洗等问题。
python爬虫爬取懂车帝网页数据
要使用Python爬虫来爬取懂车帝网页数据,你可以按照以下步骤进行操作:
1. 首先,你需要导入所需的库和模块。常用的库包括requests和beautifulsoup4,用于发送HTTP请求和解析HTML页面。你还可以使用其他库来处理数据和存储结果,如pandas或sqlite3。
2. 接下来,你需要发送HTTP请求获取懂车帝网页的内容。可以使用requests库的get方法来发送GET请求,并指定目标URL。通过观察懂车帝网页的结构,你可以确定要爬取的数据在哪个URL上。
3. 一旦获取了网页的内容,你可以使用beautifulsoup4库来解析HTML页面。使用beautifulsoup4的select或find方法选择要提取的数据所在的HTML元素。根据引用,你可以使用json()方法来解析返回的JSON数据。
4. 如果需要登录或在请求中使用特定的header来绕过反爬措施,可以参考引用中提到的添加header的方法。
5. 解析和提取数据后,你可以根据需要进行处理和分析。你可以使用pandas库将数据存储为DataFrame,并进行进一步的数据分析。如果你希望将数据存储在数据库中,可以使用sqlite3库或其他适合的数据库库。
综上所述,你可以使用Python的爬虫技术来爬取懂车帝网页数据。首先发送HTTP请求获取网页内容,然后使用beautifulsoup4库解析和提取所需的数据。最后,你可以使用pandas库进行数据处理和分析,或使用sqlite3库将数据存储在数据库中。希望这些步骤对你有帮助。
相关推荐
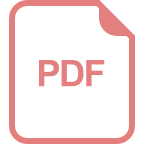
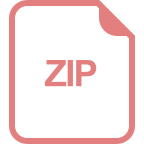









