c语言编写单词搜索软件
时间: 2023-08-10 18:09:37 浏览: 84
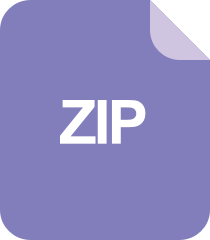
c语言编写软件
以下是一个简单的 C 语言代码示例,用于在给定的文本中搜索给定单词:
```c
#include <stdio.h>
#include <string.h>
#define MAX_WORD_LEN 50
#define MAX_TEXT_LEN 1000
int main() {
char word[MAX_WORD_LEN], text[MAX_TEXT_LEN];
int word_len, text_len, i, j, found;
printf("Enter a word to search for: ");
fgets(word, MAX_WORD_LEN, stdin);
word[strcspn(word, "\n")] = '\0'; // remove newline character
printf("Enter the text to search in: ");
fgets(text, MAX_TEXT_LEN, stdin);
text[strcspn(text, "\n")] = '\0'; // remove newline character
word_len = strlen(word);
text_len = strlen(text);
found = 0;
for (i = 0; i < text_len - word_len + 1; i++) {
for (j = 0; j < word_len; j++) {
if (text[i+j] != word[j]) {
break;
}
}
if (j == word_len) { // word found
found = 1;
printf("'%s' found at position %d.\n", word, i+1);
}
}
if (!found) {
printf("'%s' not found.\n", word);
}
return 0;
}
```
这个程序首先要求用户输入要搜索的单词和要搜索的文本,然后使用两个嵌套的循环逐个比较文本中的字符和单词中的字符来查找单词。如果找到单词,则输出其位置;否则输出未找到的消息。请注意,此代码示例仅适用于简单的搜索,无法处理大小写、标点符号等问题。如果需要更复杂的搜索功能,需要进行更多的代码编写。
阅读全文
相关推荐
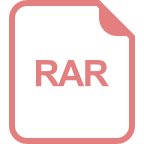

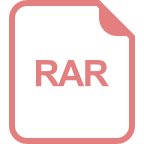
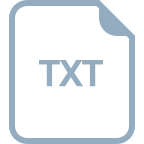
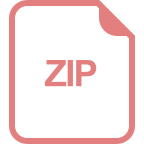
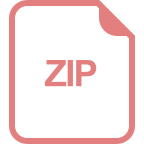
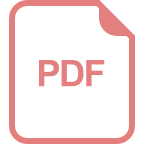
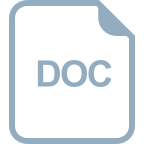
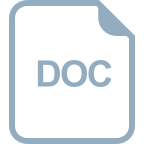





